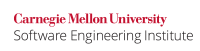
Different system architectures use different byte ordering, either little endian (least significant bit first) or big endian (most significant byte first). IA_32 is an example of an architecture that implements little endian byte ordering. PowerPC and most Network Protocols (including TCP and IP) use big endian.
When transferring data between systems of different endianness, the programmer must take care to reverse the byte ordering before they interpret the data.
Noncompliant Code Example
In this non-compliant code example, the programmer tries to read an integer off a previously connected network socket: (assume 32 bit little endian system)
/*sock is a connected TCP socket*/ int num; if(recv(sock,(void*)&num,sizeof(int),0) <0){ /*handle error*/ } printf("We received %d from the network!\n",num);
This program will print out the number received off the socket in the incorrect byte ordering, therefore (assuming an x86 system), if the number 4 was sent, the number 536 870 912 will be read. To remediate this, the programmer must reverse the byte ordering:
Compliant Code Example
In this compliant code example, the programmer correctly utilizes the ntohl function to convert the integer from network byte order to host byte ordering.
/*sock is a connected TCP socket*/ int num; if(recv(sock,(void *)&num,sizeof(int),0)<0){ /*handle error*/ } num = ntohl(num); printf("We recieved %d from the network!\n",num);
The ntohl (network to host long) translates a long type (which is the same size as an int), into the host byte ordering from the network byte ordering. This function is always appropiate to use since it is implementation defined on systems per that systems byte ordering. Thus on a PowerPC system, ntohl does nothing.
The similar function htonl (host to network long) should be used before sending any data to another system over network protocols.
Risk Assessment
If the programmer is careless then this bug is very likely. However it will immediately break the program by printing the incorrect result and therefore should be caught by the programmer during the early stages of debugging and testing. Recognizing a value as in reversed byte ordering, however, can be difficult depending on the type and magnitude of the data.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
POS39-C |
high |
unlikely |
low |
P3 |
L3 |
References
POSIX ntohl man page
FIO09-C. Be careful with binary data when transferring data across systems