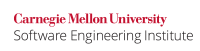
Bit-fields allow for a simple way to break portions of a struct
into portions that only use up a certain specified number of bits. If multiple threads are accessing or making modifications to different bit-fields, a race condition may be present because the architecture may not be able to modify only the bits to which the currently being modified member may refer. Therefore, a mutex protecting all bit-fields at the same time must be used.
Non-Compliant Code Example
In the following non-compliant code, two threads presumed to be running simultaneously access two separate members of a global struct
.
struct multi_threaded_flags { int flag1 : 2; int flag2 : 2; }; struct multi_threaded_flags flags; void thread1() { flags.flag1 = 1; } void thread2() { flags.flag2 = 2; }
Although this appears to be harmless, it is possible (and likely) that the architecture that this is running on has aligned flag1
and flag2
on the same byte. If both assignments occur on a thread scheduling interleaving which ends with the both stores occurring after one another, it is possible that only one of the flags will be set as intended and the other flag will equal its previous value due to the fact that both of the bit-fields fell on the same byte, which was the smallest unit the processor could work on.
Compliant Solution
This compliant solution protects all usage of the flags with a mutex, preventing an unfortunate thread scheduling interleaving from being able to occur.
struct multi_threaded_flags { int flag1 : 2; int flag2 : 2; pthread_mutex_t mutex; }; struct multi_threaded_flags flags; void thread1() { pthread_mutex_lock(&flags.mutex); flags.flag1 = 1; pthread_mutex_unlock(&flags.mutex); } void thread2() { pthread_mutex_lock(&flags.mutex); flags.flag2 = 2; pthread_mutex_unlock(&flags.mutex); }
Risk Assessment
Although the race window is very narrow, having an assignment or an expression evaluate improperly due to misinterpreted data can cause a program to misbehave, possibly resulting in a corrupted running state.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
POS32-C |
2 (medium) |
2 (probable) |
2 (medium) |
P8 |
L2 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999:TC2]] Section 6.7.2.1, "Structure and union specifiers"