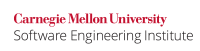
Different alignments are possible for different types of objects. If the type-checking system is overridden by an explicit cast or the pointer is converted to a void pointer (void *
) and then to a different type, the alignment of an object may be changed. As a result, if a pointer to one object type is converted to a pointer to a different object type, the second object type must not require stricter alignment than the first.
Noncompliant Code Example
C99 (and C90) allows a pointer to be cast into and out of void *
. As a result, it is possible to silently convert from one pointer type to another without the compiler diagnosing the problem by storing or casting a pointer to void *
and then storing or casting it to the final type. In this noncompliant code example, the type checking system is circumvented due to the caveats of void
pointers.
char *loop_ptr; int *int_ptr; int *loop_function(void *v_pointer) { /* ... */ return v_pointer; } int_ptr = loop_function(loop_ptr);
This example compiles without warning. However, v_pointer
may be aligned on a one-byte boundary. Once it is cast to an int *
, some architectures will require that the object is aligned on a four-byte boundary. If int_ptr
is later dereferenced, the program may terminate abnormally.
One solution is to ensure that loop_ptr
points to an object returned by malloc()
because this object is guaranteed to be aligned properly for any need. However, this is a subtlety that is easily missed when the program is modified in the future. It is cleaner to let the type system document the alignment needs.
Compliant Solution
Because the input parameter directly influences the return value, and loop_function()
returns an int *
, the formal parameter v_pointer
is redeclared to only accept int *
.
int *loop_ptr; int *int_ptr; int *loop_function(int *v_pointer) { /* ... */ return v_pointer; } int_ptr = loop_function(loop_ptr);
Risk Assessment
Accessing a pointer or an object that is no longer on the correct access boundary can cause a program to crash or give wrong information, or may cause slow pointer accesses (if the architecture allows misaligned accesses).
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP36-C |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
The LDRA tool suite V 7.6.0 can detect violations of this rule.
GCC Compiler can detect some violations of this rule when the -Wcast-align
flag is used.
Compass/ROSE could detect violations of this rule by checking for pointer casts where the new object size is smaller than the old object size.
bigtype bt; smalltype *ptr = (smalltype*) (&bt);
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Bryant 03]]
[[ISO/IEC 9899:1999]] Section 6.2.5, "Types"
[[ISO/IEC PDTR 24772]] "HFC Pointer casting and pointer type changes"
[[MISRA 04]] Rules 11.2 and 11.3
EXP35-C. Do not access or modify an array in the result of a function call after a subsequent sequence point 03. Expressions (EXP) EXP37-C. Call functions with the arguments intended by the API