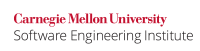
Typically, there are several different possible alignments used for the fundamental types of C. If the C type checking system is overridden by an explicit cast, it is possible the alignment of the underlying object or type may not match up with the object to which it was cast. Therefore, the alignment must always be the same if a pointer is to be cast into another.
Non-compliant Code Example
By definition of C99, a pointer may be cast into and out of void*
validly. Thus it is possible to silently switch from one type of pointer to another without flagging a compiler warning by first storing or casting the initial pointer to void*
and then storing or casting it to the final type. In the following non-compliant code, the type checking system is circumvented due to the caveats of void
pointers.
char *loop_ptr; int *int_ptr; int *loop_function(void *v_pointer){ return v_pointer; } int_ptr = loop_function(loop_ptr);
This example should compile without warning. However, v_pointer
might be aligned on a 1 byte boundary. Once it is cast to an int
, some architectures will require it to be on 4 byte boundaries. If int_ptr
is then later dereferenced, abnormal termination of the program may result.
Compliant Solution
In this compliant solution, the parameter is changed to only accept other int*
pointers since the input parameter directly influences the output parameter.
int *loop_ptr; int * int_ptr; int *loopFunction(int *v_pointer) { return v_pointer; } int_ptr = loopFunction(loop_ptr);
Implementation Details
List of common alignments for Microsoft, Borland, and GNU compilers to x86
Type |
Alignment |
---|---|
|
1 byte aligned |
|
2 byte aligned |
|
4 byte aligned |
|
4 byte aligned |
|
8 byte on Windows, 4 byte on Linux |
Risk Assessment
Accessing a pointer or an object that is no longer on the correct access boundary can cause a program to crash, give wrong information, or may cause slow pointer accesses (if the architecture does not care about alignment).
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP36-C |
1 (low) |
2 (probable) |
2 (medium) |
P4 |
L3 |
References
[[Bryant 03]]
[[ISO/IEC 9899-1999:TC2]] Section 6.2.5, "Types"