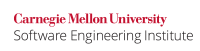
Functions should provide a consistent and easy to use interface for error checking. Complex interfaces are sometimes ignored by programmers, resulting in code that is not error checked. Inconsistent interfaces are frequently misused and difficult to use, resulting in lower quality code and higher development costs.
Noncompliant Code Example (strlcpy()
)
The standard strncpy()
function does not guarantee that the resulting string is null terminated [[ISO/IEC 9899:1999]]. If there is no null character in the first n
characters of the source
array, the result may not be null terminated.
char *source; char a[NTBS_SIZE]; /* ... */ if (source) { errno_t err = strncpy(a, source, 5); if (err != 0) { /* Handle error */ } } else { /* handle NULL string condition */ }
Compliant Solution (strcpy_m()
)
The managed string library handles errors by consistently returning the status code in the function return value. This approach encourages status checking because the user can insert the function call as the expression in an if statement and take appropriate action on failure. The greatest disadvantage of this approach is that it prevents functions from returning any other value. This means that all values (other than the status) returned by a function must be returned as a pass-by-reference parameter, preventing a programmer from nesting function calls. This tradeoff is necessary because nesting function calls can conflict with a programmer's willingness to check status codes.
errno_t retValue; char *cstr; // c style string string_m str1 = NULL; if (retValue = strcreate_m(&str1, "hello, world", 0, NULL)) { fprintf(stderr, "Error %d from strcreate_m.\n", retValue); } else { /* handle NULL string condition */ }
Risk Assessment
Failure to do so can result in type errors in the program.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
API07-C |
medium |
unlikely |
medium |
P2 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as API07-CPP. Enforce type safety.
References
[[ISO/IEC 9899:1999]] Section 7.21, "String handling <string.h>"
[[ISO/IEC PDTR 24772]] "CJM String Termination"
[[ISO/IEC TR 24731-1:2007]] Section 6.7.1.4, "The strncpy_s function"
01. Preprocessor (PRE) 02. Declarations and Initialization (DCL)