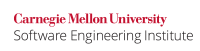
To ensure predictable program execution casts to type float must be present on all floating-point function calls.
The C99 standard [[ISO/IEC 9899:1999]] states in Section 6.8.6.4, Paragraph 3,
If a return statement with an expression is executed, the value of the expression is returned to the caller as the value of the function call expression. If the expression has a type different from the return type of the function in which it appears, the value is converted as if by assignment to an object having the return type of the function. 142)
This paragraph is footnoted as follows:
142) The return statement is not an assignment. The overlap restriction of subclause 6.5.16.1 does not apply to the case of function return. The representation of floating-point values may have wider range or precision. A cast may be used to remove this extra range and precision.
Conversion as if by assignment to the type of the function is required if the return expression has a different type than the function, but not if the return expression has a wider value only because of wide evaluation. This allows seemingly inconsistent and confusing behavior. Consider,
float f(float x) { Â return x * 0.1f; } float g(float x) { Â return x * 0.1; }
Function f is allowed to return a value wider than float, but function g (which uses the wider constant) is not.
Although the current text does not require narrowing return expressions of the same type as the function, it does not clearly state what is allowed. Is it allowed to narrow the result? Is it allowed to narrow the result sometimes but not always? Is it allowed to partially narrow the result (e.g., if the ABI returns floats in double format but a float function has a float return expression evaluated to wider than double)? An aggressive implementation could argue âyesâ to all these, though the resulting behavior would complicate debugging and error analysis.
Footnote 142 says a cast may be used to remove extra range and precision from the return expression. This means a predictable program must have casts on all floating-point function calls (except where the function directly feeds an operator like assignment that implies the conversion). With type-generic math (tgmath), the programmer has to reason through the tgmath resolution rules to determine which casts to apply. These are significant obstacles to writing predictable code.
NOTE: WG14 has voted to include the following text in C1X. This only impacts implementations that implement the optional Annex F "IEC 60559 floating-point arithmetic".
Require return expressions to be converted as if by assignment to the type of the function, but only in Annex F. This is a compromise that addresses the problems for Annex F implementations while not impacting non-Annex F implementations that exercise the license for wide returns.
Insert the following new subclause after F.5 (and increment subsequent subclause numbers):
F.6 The return statement
If the return expression is evaluated in a floating-point format different from the return type, then the expression is converted to the return type of the function and the resulting value is returned to the caller.
Noncompliant Code Example
The following example code has been constructed to illustrate an example that does not conform to this recommendation. The code is non-conforming because it does not cast the result of the expression in the return statement and thereby guarantee the range or precision is no wider than expected. The uncertainty in this example is introduced by the constant 0.1f. This constant may be stored with a range or precision that is greater than that of float. Consequently, the result of x * 0.1f may also have a range or precision greater than that of float. As described above, this range or precision may not be reduced to that of a float and, thus, the caller of calcPercentage() may receive a value that is more precise than expected. This may lead to inconsistent program execution across different platforms.
float calcPercentage(float value) { Â return value * 0.1f; } void floatRoutine(void) { float value = 99.0f; long double percentage; percentage = calcPercentage(value); }
Compliant Code Example
The following code example remedies the above noncompliant code by casting the value of the expression in the return statement. This forces the return value to have the expected range and precision as described in Section 5.2.4.2.2 8 of the C Standard.
float calcPercentage(float value) { Â return (float)(value * 0.1f); } void floatRoutine(void) { float value = 99.0f; long double percentage; percentage = calcPercentage(value); }
Compliant Code Example (Alternate)
Unfortunately, not all compilers may honor casts. In this case the range and precision must be forced by assignment to a variable of the correct type. The following code example illustrates this approach.
float calcPercentage(float value) { volatile float result; result = value * 0.1f; Â return result; } void floatRoutine(void) { float value = 99.0f; long double percentage; percentage = calcPercentage(value); }
Compliant Code Example (Outside the function 1)
Forcing the range and precision inside the calcPercentage() function is a good way to fix the problem once without having to apply fixes in multiple locations (every time calcPercentage() is called). However, access to the called function may not always be available. This example shows one way to force the correct range and precision in a situation in which the source of the called function can not be modified. This is accomplished by casting the return value of the calcPercentage() function to float.
void floatRoutine(void) { float value = 99.0f; long double percentage; percentage = (float)calcPercentage(value); }
Compliant Code Example (Outside the function 2)
This example shows another way to force the correct range and precision. In this case a temporary variable is used as the forcing mechanism.
void floatRoutine(void) { float value = 99.0f; long double percentage; float temp; percentage = temp = calcPercentage(value); }
Risk Assessment
Failure to follow this guideline can lead to inconsistent results across different platforms.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FLP37-C |
low |
unlikely |
medium |
P2 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]]
[[WG14/N1396]]