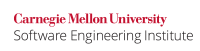
Code that is executed but does not perform any action, or that has an unintended effect, most likely results from a coding error and can cause unexpected behavior. Statements or expressions that have no effect should be identified and removed from code. Most modern compilers, in many cases, can warn about code that has no effect. (See MSC00-C. Compile cleanly at high warning levels.)
This recommendation is related to MSC07-C. Detect and remove dead code.
Noncompliant Code Example (Assignment)
In this noncompliant code example, the comparison of a
to b
has no effect:
int a; int b; /* ... */ a == b;
This code is likely a case of the programmer mistakenly using the equals operator ==
instead of the assignment operator =
.
Compliant Solution (Assignment)
The assignment of b
to a
is now properly performed:
int a; int b; /* ... */ a = b;
Noncompliant Code Example (Dereference)
In this example, a pointer increment and then a dereference occur, but the dereference has no effect:
int *p; /* ... */ *p++;
Compliant Solution (Dereference)
Correcting this example depends on the intent of the programmer. For example, if dereferencing p
was a mistake, then p
should not be dereferenced.
int *p; /* ... */ ++p;
If the intent was to increment the value referred to by p
, then parentheses can be used to ensure p
is dereferenced and then incremented. (See EXP00-C. Use parentheses for precedence of operation.)
int *p; /* ... */ (*p)++;
Compliant Solution (Memory-Mapped Devices)
Another possibility is that p
is being used to reference a memory-mapped device. In this case, the variable p
should be declared as volatile
.
volatile int *p; /* ... */ (void) *(p++);
Noncompliant Code Example (if/else if)
A chain of if/else if statements is evaluated from top to bottom. At most, only one branch of the chain will be executed: the first one with a condition that evaluates to true. Consequently, duplicating a condition in a sequence of if/else if statements automatically leads to dead code.
if (param == 1) openWindow(); else if (param == 2) closeWindow(); else if (param == 1) /* Duplicated condition */ moveWindowToTheBackground();
Compliant Solution (if/else if)
In this compliant solution, the third conditional expression has been corrected.
if (param == 1) openWindow(); else if (param == 2) closeWindow(); else if (param == 3) moveWindowToTheBackground();
Noncompliant Code Example (logical operators)
Using the same subexpression on either side of a logical operator is almost always a mistake. In this noncompliant code example, the rightmost subexpression of the controlling expression of each if
statement has no effect.
if (a == b && a == b) { // if the first one is true, the second one is too do_x(); } if (a == c || a == c ) { // if the first one is true, the second one is too do_w(); }
Compliant Solution (logical operators)
In this compliant solution, the rightmost subexpression of the controlling expression of each if
statement has been removed.
if (a == b) { do_x(); } if (a == c) { do_w(); }
Risk Assessment
The presence of code that has no effect can indicate logic errors that may result in unexpected behavior and vulnerabilities.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC12-C | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 8.1p0 | LANG.STRUCT.EBS LANG.STRUCT.RC MISC.NOEFFECT | Empty {Branch, for, if, switch, while} Statement Redundant Condition Function Call Has No Effect |
2017.07 | NO_EFFECT | Finds statements or expressions that do not accomplish anything or statements that perform an unintended action | |
1.2 | CC2.MSC12 | Partially implemented | |
3.0 | Options detect unused local variables or nonconstant static variables and unused function parameters, respectively | ||
2024.1 | EFFECT |
| |
9.7.1 | 65 D | Fully implemented | |
PRQA QA-C | Unable to render {include} The included page could not be found. | 3426,3427,3307,3110,3112,3404 | Partially implemented |
SonarQube Plugin | Unable to render {include} The included page could not be found. | S1862, S1764, S1751, S1116, S1656 | |
3.1.1 |
|
|
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
CERT C++ Coding Standard | MSC12-CPP. Detect and remove code that has no effect |
ISO/IEC TR 24772 | Unspecified Functionality [BVQ] Likely Incorrect Expressions [KOA] Dead and Deactivated Code [XYQ] |
MISRA C:2012 | Rule 2.2 (required) |
Bibliography