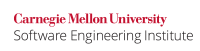
The functions in the C standard library are not guaranteed to be reentrant with respect to threads. Some functions return a pointer to the result stored in memory allocated by the function on a per-process basis(such as strtok() and asctime()) while some functions store state information in memory allocated by the function on a per-process basis(such as rand()). Multiple threads invoking the same function can cause concurrency problems. Concurrency problems can often result in abnormal behavior, but it is possible for them to result in more serious vulnerabilities such as abnormal termination, denial of service attack and data integrity violation.
As per the N1401-C1X document, the following library functions are not required to avoid data races
- rand()
- getenv()
- strtok()
- strerror()
- asctime()
- ctime()
Non Compliant Code
Consider a multithreaded application which involves a function which returns a random value each time it is invoked. According to POSIX implementation, rand() returns the next pseudorandom number in the sequence determined by an initial seed value. The rand() function updates the seed value, which is stored at a library allocated static memory location. If two threads concurrently invoke the rand() function, it may result in undefined behavior and may also result in rand() returning the same value in both the threads.
int get_secret() { int secret = (rand() % 100) + 100; return secret; }
Compliant Solution
The compliant solution uses a mutex to make each call to rand() function atomic.
#include <pthread.h> pthread_mutex_t rand_lock = PTHREAD_MUTEX_INITIALIZER; int get_secret() { int secret; pthread_mutex_lock(&rand_lock) ; secret = (rand() % 100) + 100; pthread_mutex_unlock(&rand_lock); return secret; }
Risk Assessment
Race conditions caused by multiple threads invoking the same library function can lead to abnormal termination of the application, data integrity violations or denial of service attack.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
C1X00-C |
medium |
probable |
High |
P4 |
L3 |
References
[N1401-C1X Draft] Section 7.21.2.1 rand() function, Section 7.21.4.6 getenv() function, Section 7.22.5.8 strtok() function, Section 7.22.6.2 strerror() function, Section 7.25.3.1 asctime() function, Section 7.25.3.2 ctime() function
[POSIX.1 Thread Safety]