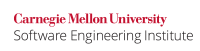
Mutexes are used to protect shared data structures being accessed concurrently. The thread that locks the mutex owns it, and the owning thread should be the only thread to unlock the mutex. If the mutex is destroyed while still in use, critical sections and shared data are no longer protected. This rule is a specific instance of CON31-C. Do not unlock or destroy another thread's mutex using POSIX threads.
Noncompliant Code Example
In this noncompliant code example, a race condition exists between a cleanup and a worker thread. The cleanup thread destroys the lock, which it believes is no longer in use. If there is a heavy load on the system, the worker thread that held the lock can take longer than expected. If the lock is destroyed before the worker thread has completed modifying the shared data, the program may exhibit unexpected behavior.
pthread_mutex_t theLock; int data; int cleanupAndFinish(void) { int result; if ((result = pthread_mutex_destroy(&theLock)) != 0) { /* Handle error */ } data++; return data; } void worker(int value) { if ((result = pthread_mutex_lock(&theLock)) != 0) { /* Handle error */ } data += value; if ((result = pthread_mutex_unlock(&theLock)) != 0) { /* Handle error */ } }
Compliant Solution
This compliant solution requires that there is no chance a mutex will be needed after it has been destroyed. As always, it is important to check for error conditions when locking the mutex.
mutex_t theLock; int data; int cleanupAndFinish(void) { int result; /* A user-written function that is application-dependent */ wait_for_all_threads_to_finish(); if ((result = pthread_mutex_destroy(&theLock)) != 0) { /* Handle error */ } data++; return data; } void worker(int value) { int result; if ((result = pthread_mutex_lock(&theLock)) != 0) { /* Handle error */ } data += value; if ((result = pthread_mutex_unlock(&theLock)) != 0) { /* Handle error */ } }
Risk Assessment
The risks of ignoring mutex ownership are similar to the risk of not using mutexes at all, which can result in a violation of data integrity.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
POS48-C | medium | probable | high | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Fortify SCA | V. 5.0 | Can detect violations of this rule with CERT C Rule Pack. |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
Bibliography