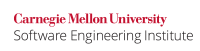
No direct issues come from using the same handler for multiple signals, but it broadens your susceptibility to other vulnerabilities. For instance, if a signal handler is constructed with the expectation that it will only be executed once, but the handler is registered to catch multiple signals, then it may perform an operation multiple times that should only be performed once. Depending on the handler, this may provide a means to exploit other vulnerabilities. To eliminate this attack vector, each signal handler should only be registered to handle one type of signal.
Non-Compliant Coding Example
The program is intended to clean up and terminate when it receives either a SIGINT
or a SIGTERM
. However, if a SIGINT
is generated, and then a SIGTERM
is generated after the call to free()
, but before _Exit()
is reached, a double free()
will occur. Note that this example also violates SIG30-C.
#include <signal.h> char *global_ptr; void handler() { free(global_ptr); _Exit(-1); } int main() { global_ptr = malloc(16); if (global_ptr == NULL) { /* handle error condition */ } signal(SIGINT, handler); signal(SIGTERM, handler); /* program code */ return 0; }
Risk Assessment
Depending on the code, this could lead to any number of attacks, many of which could give root access. For an overview of some software vulnerabilities, see Zalewski's signal article.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SIG00-A |
3 (high) |
3 (likely) |
1 (high) |
P9 |
L2 |
References
[[ISO/IEC 03]] "Signals and Interrupts"
[[Open Group 04]] longjmp
[OpenBSD] signal()
Man Page
[Zalewski] http://lcamtuf.coredump.cx/signals.txt
[[Dowd 06 ]] Chapter 13, "Synchronization and State" (Signal Interruption and Repetition)