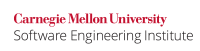
While it has been common practice to use integers and pointers interchangeably in C, pointer to integer and integer to pointer conversions are implementation-defined.
According to C99 [[ISO/IEC 9899-1999]], the only value that can be considered interchangeable between pointers and integers is the constant 0. Except in this case, conversions between integers and pointers may have undesired consequences depending on the implementation:
An integer may be converted to any pointer type. Except as previously specified, the result is implementation-defined, might not be correctly aligned, might not point to an entity of the referenced type, and might be a trap representation.
These issues arise because the mapping functions for converting a pointer to an integer or an integer to a pointer must be consistent with the addressing structure of the execution environment.
Non-Compliant Code Example
In this non-compliant code example, the pointer ptr
is used in an arithmetic operation that is eventually converted to an integer value. As previously stated, the result of this assignment and following assignment to ptr2
are implementation-defined.
unsigned int number = ptr + 1; unsigned int *ptr2 = ptr;
Compliant Solution
A union can be used to give raw memory access to both an integer and a pointer. This is an efficient approach, as the structure only requires as much storage as the larger of the two fields.
union intpoint { unsigned int *pointer; unsigned int number; } intpoint; /* ... */ intpoint mydata = 0xcfcfcfcf; /* ... */ unsigned int num = mydata.number + 1; unsigned int *ptr = mydata.pointer;
Non-Compliant Code Example
It is sometimes necessary in low level kernel or graphics code to access memory at a specific location, requiring a literal integer to pointer to conversion. In this non-compliant code, a pointer is set directly to an integer constant.
unsigned int *ptr = 0xcfcfcfcf;
The result of this assignment is implementation-defined, might not be correctly aligned, might not point to an entity of the referenced type, and might be a trap representation.
Compliant Solution
Adding an explicit cast may help the compiler convert the integer value into a valid pointer.
unsigned int *ptr = (unsigned int *)0xcfcfcfcf;
Risk Analysis
Converting from pointer to integer or vice versa results in unportable code and may create unexpected pointers to invalid memory locations.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT15-A |
1 (low) |
2 (probable) |
1 (high) |
P2 |
L3 |
References
[[ISO/IEC 9899-1999]] Section 6.3.2.3, "Pointers"