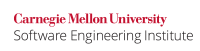
Wide characters may frequently contain null bytes if taken from the ASCII character set. As a result, using narrow-char functions which rely on null-byte termination may lead to obtuse behavior. Likewise, a narrow-char string which is properly null-terminated may not be considered so in a wide-char function. Improper use of narrow and wide character strings could result in buffer overflows.
Noncompliant Code Example (Using strncpy Instead of wcsncpy)
The below example uses strncpy
which will copy at most 10 bytes in this example, but will stop copying after it encounters a null-byte. Since wide-characters may contain null-bytes, it may stop copying prematurely. It is important to recognize that many narrow-string functions are byte functions, and, thus, may terminate prematurely.
wchar_t *wide_str1 = L"0123456789"; wchar_t *wide_str2 = L"0000000000"; strncpy(wide_str2, wide_str1, 10);
Noncompliant Code Example (Using wcsncpy Instead of strncpy)
The below example uses wcsncpy
, which will copy 10 wide-length characters. In most implementations, wide-characters span multiple narrow-characters. The wcsncpy
function will copy at most 10 wide-characters, which is longer than narrow_str1
. As a result, it will write the first 10 bytes of narrow_str1
into narrow_str2
and then continue padding with L'\0'
null wide-characters until 10 wide-characters have been written.
It should be noted that wcsncpy
does not perform null-termination if the source string contains more wide-characters than the destination. As a result, it is possible for an attacker to exploit such a vulnerability by passing a maliciously crafted string to wcsncpy
. If the code is intended to copy a certain number of bytes, it may overflow the buffer by writing multiple bytes as wcsncpy
measures copying by wide-characters, not by bytes.
char *narrow_str1 = "0123456789"; char *narrow_str2 = "0000000000"; wcsncpy(narrow_str2, narrow_str1, 10);
Implementation Details
C99 recognizes wchar_t *
and char *
as distinct types. As a result, many compilers will yield a warning if the inappropriate function is used. For example, the following warnings were generated when the second non-compliant example was compiled with no flags in GCC on a Linux i686 platform:
warning: passing arg 1 of `wcsncpy' from incompatible pointer type warning: passing arg 2 of `wcsncpy' from incompatible pointer type
Similar warnings were issued by the compiler for the first non-compliant example, with respect to the arguments of the strncpy
function instead.
Since these are just warnings, the compiled code can still be run. When run on the i686 Linux platform mentioned above, both noncompliant code examples began copying information from out of the bounds of the arguments. This behavior is indicative a possible buffer overflow vulnerability.
Compliant Solution
The below example uses the appropriate-width function versions. Using wcsncpy
for wide-char strings and strncpy
for narrow-char strings will ensure that data is not truncated or overwriting extra memory.
wchar_t *wide_str1 = L"0123456789"; wchar_t *wide_str2 = L"0000000000"; wcsncpy(wide_str2, wide_str1, 10); /* Use of proper-width function */ char *narrow_str1 = "0123456789"; char *narrow_str2 = "0000000000"; strncpy(narrow_str2, narrow_str1, 10); /* Use of proper-width function */
Risk Assessment
Failure to use the proper-width string functions can lead to buffer overflows and the execution of arbitrary code by an attacker.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
STR38-C |
high |
likely |
low |
P27 |
L1 |
Automated Detection
Modern compilers recognize the difference between a char*
and a wchar_t*
pointer. As a result, compiling code that violates this rule will generate warnings. It is feasible to have automated software that recognizes improper-width functions and replaces them with their proper width functions (i.e., using wcsncpy
when it recognizes that the parameters are of type wchar_t*
).
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Bibliography
[ISO/IEC 9899:1999] Section 7.21.2.4, "The strncpy
function"
[ISO/IEC 9899:1999] Section 7.24.4.2.2, "The wcsncpy
function"
STR37-C. Arguments to character handling functions must be representable as an unsigned char 07. Characters and Strings (STR) 08. Memory Management (MEM)