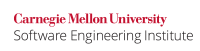
Although many common implementations use a two's complement representation of signed integers, the C99 standard declares this as implementation-defined, and allows all of the following representations:
- Sign and magnitude
- Two's complement
- Ones' complement
This is a specific example of the recommendation MSC14-C. Do not introduce unnecessary platform dependencies.
Noncompliant Code Example
One way to check whether a number is even or odd is to examine the least significant bit. This will give inconsistent results. Specifically, this example will give unexpected behavior on all ones' complement implementations.
int value; if (scanf("%d", &value) == 1) { if (value & 0x1 == 1) { /* do something if value is odd */ } }
Compliant Solution
The same thing can be achieved compliantly using the modulo operator.
int value; if (scanf("%d", &value) == 1) { if (value % 2 == 1) { /* do something if value is odd */ } }
Risk Assessment
Incorrect assumptions about integer representation can lead to execution of unintended code branches and other unexpected behavior.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT16-C |
medium |
unlikely |
high |
P6 |
L2 |
Bibliography
[ISO/IEC 9899:1999] Section 6.2.6.2