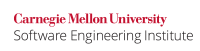
Section 7.19.5.3 of C99 places the following restrictions on update streams [ISO/IEC 9899:1999]:
When a file is opened with update mode both input and output may be performed on the associated stream. However, output shall not be directly followed by input without an intervening call to the
fflush
function or to a file positioning function (fseek
,fsetpos
, orrewind
), and input shall not be directly followed by output without an intervening call to a file positioning function, unless the input operation encounters end-of-file. Opening (or creating) a text file with update mode may instead open (or create) a binary stream in some implementations.
Receiving input from a stream directly following an output to that stream without an intervening call to fflush()
, fseek()
, fsetpos()
, or rewind()
, or outputting to a stream after receiving input from it without a call to fseek()
, fsetpos()
, rewind()
if the file is not at end-of-file results in undefined behavior. (See also undefined behavior 143 in Annex J of C99.) Consequently, a call to fseek()
, fflush()
or fsetpos()
is necessary between input and output to the same stream. (See recommendation FIO07-C. Prefer fseek() to rewind().)
Noncompliant Code Example
This noncompliant code example appends data to a file and then reads from the same file.
char data[BUFFERSIZE]; char append_data[BUFFERSIZE]; char *file_name; FILE *file; /* Initialize file_name */ file = fopen(file_name, "a+"); if (file == NULL) { /* Handle error */ } /* initialize append_data */ if (fwrite(append_data, BUFFERSIZE, 1, file) != BUFFERSIZE) { /* Handle error */ } if (fread(data, BUFFERSIZE, 1, file) != 0) { /* Handle there not being data */ } fclose(file);
However, because the stream is not flushed in between the call to fread()
and fwrite()
, the behavior is undefined.
Compliant Solution
In this compliant solution, fseek()
is called in between the output and input, eliminating the undefined behavior.
char data[BUFFERSIZE]; char append_data[BUFFERSIZE]; char *file_name; FILE *file; /* initialize file_name */ file = fopen(file_name, "a+"); if (file == NULL) { /* Handle error */ } /* Initialize append_data */ if (fwrite(append_data, BUFFERSIZE, 1, file) != BUFFERSIZE) { /* Handle error */ } if (fseek(file, 0L, SEEK_SET) != 0) { /* Handle error */ } if (fread(data, BUFFERSIZE, 1, file) != 0) { /* Handle there not being data */ } fclose(file);
Risk Assessment
Alternately inputting and outputting from a stream without an intervening flush or positioning call results in undefined behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO39-C |
low |
likely |
medium |
P6 |
L2 |
Automated Detection
Tool |
Version |
Checker |
Description |
---|---|---|---|
Fortify SCA |
V. 5.0 |
|
can detect violations of this rule with CERT C Rule Pack |
Compass/ROSE |
|
|
can detect simple violations of this rule |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
CERT C++ Secure Coding Standard: FIO39-CPP. Do not alternately input and output from a stream without an intervening flush or positioning call
ISO/IEC 9899:1999 Section 7.19.5.3, "The fopen
function"
Bibliography
FIO38-C. Do not use a copy of a FILE object for input and output 09. Input Output (FIO) FIO40-C. Reset strings on fgets() failure