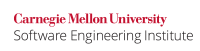
The C99 fopen()
function has the capability to open files to which both input and output are capable. This functionality is known as "update" mode and is signified by using '+
' as the second or third character in the mode string to fopen()
.
However, section 7.19.5.3 of C99 places the following restriction on update streams:
When a file is opened with update mode ('
+
' as the second or third character in the above list of mode argument values), both input and output may be performed on the associated stream. However, output shall not be directly followed by input without an intervening call to thefflush
function or to a file positioning function (fseek
,fsetpos
, orrewind
), and input shall not be directly followed by output without an intervening call to a file positioning function, unless the input operation encounters end-of-file. Opening (or creating) a text file with update mode may instead open (or create) a binary stream in some implementations.
Therefore either receiving input from a stream directly following an output to that stream without an intervening call to fflush()
, fseek()
, fsetpos()
, or rewind()
, or outputting to a stream after receiving input from it without a call to fseek()
, fsetpos()
, rewind()
if the file is not at end-of-file results in undefined behavior. Consequently a call to one of these functions is necessary in between input and output to the same stream in most cases.
Non-Compliant Code Example
The following non-compliant code reads data from a presumably random area of a file, and then appends data to the end of it.
char data[BUF_SIZE]; char append_data[BUF_SIZE]; size_t append_size; FILE *file = fopen(file_name, "a+"); if (file == NULL) { /* handle error */ } if (fread(data, BUF_SIZ, 1, file) != 0) { /* Handle there not being data */ } /* Do something with data Initialize append_data and append_size */ if(fwrite(append_data, append_size, 1, data) != append_size) { /* Handle error */ } fclose(file);
However, since the stream is not flushed in between the call to fread()
and fwrite()
, undefined behavior results.
Compliant Solution
In this compliant solution, fflush()
is called in between the output and input, removing the undefined behavior.
char data[BUF_SIZE]; char append_data[BUF_SIZE]; size_t append_size; FILE *file = fopen(file_name, "a+"); if (file == NULL) { /* handle error */ } if (fread(data, BUF_SIZ, 1, file) != 0) { /* Handle there not being data */ } /* Do something with data Initialize append_data and append_size */ fflush(file); if(fwrite(append_data, append_size, 1, data) != append_size) { /* Handle error */ } fclose(file);
Risk Assessment
TODO, causing unexpected program behavior and possibly a data integrity violation.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO39-C |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
Fortify SCA Version 5.0 with CERT C Rule Pack can detect violations of this rule.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]] Section 7.19.5.3, "The fopen
function"
FIO38-C. Do not use a copy of a FILE object for input and output 09. Input Output (FIO) FIO40-C. Reset strings on fgets() failure