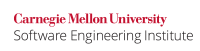
If a floating-point value is to be demoted to a floating-point value of a smaller range and precision or to an integer type, or if an integer type is to be converted to a floating-point type, the value must be represented in the new type.
Subclause 6.3.1.4 of the C Standard [ISO/IEC 9899:2011] says,
When a finite value of real floating type is converted to an integer type other than
_Bool
, the fractional part is discarded (i.e., the value is truncated toward zero). If the value of the integral part cannot be represented by the integer type, the behavior is undefined.When a value of integer type is converted to a real floating type, if the value being converted can be represented exactly in the new type, it is unchanged. If the value being converted is in the range of values that can be represented but cannot be represented exactly, the result is either the nearest higher or nearest lower representable value, chosen in an implementation-defined manner. If the value being converted is outside the range of values that can be represented, the behavior is undefined.
And subclause 6.3.1.5 says,
When a value of real floating type is converted to a real floating type, if the value being converted can be represented exactly in the new type, it is unchanged. If the value being converted is in the range of values that can be represented but cannot be represented exactly, the result is either the nearest higher or nearest lower representable value, chosen in an implementation-defined manner. If the value being converted is outside the range of values that can be represented, the behavior is undefined.
See also undefined behaviors 17 and 18 in Annex J of the C Standard.
Consequently, in implementations that do not allow for the representation of all numbers, conversions of numbers between zero and FLT_MIN
may result in undefined behavior.
This rule does not apply to demotions of floating-point types on implementations that support signed infinity, such as IEEE 754, as all numbers are representable.
Noncompliant Code Example (Int-Float)
This noncompliant code example leads to undefined behavior if the integral part of f_a
cannot be represented as an integer:
void func(float f_a) { int i_a; /* Undefined if the integral part of f_a > INT_MAX */ i_a = f_a; }
Compliant Solution (Int-Float)
This compliant solution assumes that the range of floating-point values is greater than that of an int
. (This is the case in almost all implementations.) Unfortunately, there is no safe way to inquire about this assumption in the code short of already knowing the implementation.
#include <limits.h> void func(float f_a) { int i_a; if (f_a > (float) INT_MAX || f_a < (float) INT_MIN) { /* Handle error */ } else { i_a = f_a; } }
Noncompliant Code Example (Demotions)
This noncompliant code example contains conversions that may be outside the range of the demoted types:
void func(double d_a, long double big_d) { double d_b = (float)big_d; float f_a = (float)d_a; float f_b = (float)big_d; }
As a result of these conversions, it is possible that d_a
is outside the range of values that can be represented by a float or that big_d
is outside the range of values that can be represented as either a float
or a double
. If this is the case, the result is undefined.
Compliant Solution (Demotions)
This compliant solution checks whether the values to be stored can be represented in the new type:
#include <float.h> void func(double d_a, long double big_d) { double d_b; float f_a; float f_b; if (d_a > FLT_MAX || d_a < -FLT_MAX) { /* Handle error condition */ } else { f_a = (float)d_a; } if (big_d > FLT_MAX || big_d < -FLT_MAX) { /* Handle error condition */ } else { f_b = (float)big_d; } if (big_d > DBL_MAX || big_d < -DBL_MAX) { /* Handle error condition */ } else { d_b = (double)big_d; } }
Risk Assessment
Failing to check that a floating-point value fits within a demoted type can result in a value too large to be represented by the new type, resulting in undefined behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
FLP34-C | Low | Unlikely | Low | P3 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
|
| Can detect some violations of this rule. However, it does not flag implicit casts, only explicit ones | |
2017.07 | MISRA_CAST | Can detect instances where implicit float conversion is involved: implicitly converting a complex expression with integer type to floating type, implicitly converting a double expression to narrower float type (may lose precision), implicitly converting a complex expression from | |
5.0 |
| Can detect violations of this rule with CERT C Rule Pack | |
PRQA QA-C | Unable to render {include} The included page could not be found. | 4450 | Partially implemented |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
CERT C++ Secure Coding Standard | FLP34-CPP. Ensure that floating point conversions are within range of the new type |
CERT Oracle Secure Coding Standard for Java | NUM12-J. Ensure conversions of numeric types to narrower types do not result in lost or misinterpreted data |
ISO/IEC TR 24772:2013 | Numeric Conversion Errors [FLC] |
MITRE CWE | CWE-681, Incorrect conversion between numeric types |
Bibliography
[IEEE 754] | IEEE 754-1985 Standard for Binary Floating-Point Arithmetic |
[ISO/IEC 9899:2011] | Subclause 6.3.1.4, "Real Floating and Integer" Subclause 6.3.1.5, "Real Floating Types" |