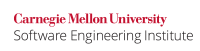
When multiple threads can read or modify the same data, use synchronization techniques to avoid software flaws that could lead to security vulnerabilities. Concurrency problems can often result in abnormal termination or denial of service, but it is possible for them to result in more serious vulnerabilities.
Non-Compliant Code Example
Assume this simplified code is part of a multithreaded bank system. Threads will call credit()
and debit()
as money is deposited into and taken from the single account. Because the addition and subtraction operations are not atomic, it is possible that two operations could occur concurrently but only the result of one would be saved. For example, an attacker could credit the account with a sum of money and make a very large number of small debits concurrently. Some of the debits might not affect the account balance because of the race condition, so the attacker is effectively creating money.
int account_balance; void debit(int amount) { account_balance -= amount; } void credit(int amount) { account_balance += amount; }
Compliant Solution
This solution uses a mutex to make credits and debits atomic operations. All credits and debits will now effect the account balance, so an attacker cannot exploit the race condition to steal money from the bank. Note that the functions used are pthread_*
. The standard mutex functions are not thread-safe. In addition, the volatile
keyword is used so prefetching will not occur.
#include <pthread.h> volatile int account_balance; pthread_mutex_t account_lock = PTHREAD_MUTEX_INITIALIZER; void debit(int amount) { pthread_mutex_lock(&account_lock); account_balance -= amount; pthread_mutex_unlock(&account_lock); } void credit(int amount) { pthread_mutex_lock(&account_lock); account_balance += amount; pthread_mutex_unlock(&account_lock); }
Risk Assessment
Race conditions caused by multiple threads concurrently accessing and modifying the same data could lead to abnormal termination and denial-of-service attacks, or data integrity violations.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
POS00-A |
2 (medium) |
2 (probable) |
1 (high) |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Dowd 06]] Chapter 13, "Synchronization and State"
[[Seacord 05]] Chapter 7, "File I/O"