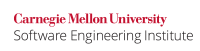
According to Section 7.4 of C99,
The header
<ctype.h>
declares several functions useful for classifying and mapping characters. In all cases the argument is anint
, the value of which shall be representable as anunsigned char
or shall equal the value of the macroEOF
. If the argument has any other value, the behavior is undefined.
Compliance with this rule is complicated by the fact that the char
data type might, in any implementation, be signed or unsigned.
The following character classification routines are affected:
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Non-Compliant Code Example
This non-compliant code example may pass invalid values to the isspace()
function.
size_t count_whitespace(char const *s, size_t length) { char const *t = s; while (isspace(*t) && (t - s < length)) /* possibly *t < 0 */ ++t; return t - s; }
Compliant Solution (Unsigned Char)
Pass character strings around explicitly using unsigned characters.
size_t count_whitespace(const unsigned char *s, size_t length) { const unsigned char *t = s; while (isspace(*t) && (t - s < length)) ++t; return t - s; }
This approach is inconvenient when you need to interwork with other functions that haven't been designed with this approach in mind, such as the string handling functions found in the standard library [[Kettlewell 02]].
Compliant Solution (Cast)
This compliant solution uses a cast.
size_t count_whitespace(char const *s, size_t length) { char const *t = s; while (isspace((unsigned char)*t) && (t - s < length)) ++t; return t - s; }
Risk Assessment
Passing values to character handling functions that cannot be represented as an unsigned char
may result in unintended program behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
STR37-C |
low |
unlikely |
low |
P3 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 7.4, "Character handling <ctype.h>"
[[Kettlewell 02]] Section 1.1, "<ctype.h> And Characters Types"
STR36-C. Do not specify the dimension of a character array initialized with a string literal 07. Characters and Strings (STR) 08. Memory Management (MEM)