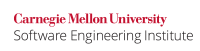
Portability is a concern when using the fread()
and fwrite()
functions across multiple, heterogeneous systems. In particular, it is never guaranteed that reading or writing of scalar data types such as integers, let alone aggregate types such as arrays or structures, will preserve the representation or value of the data. Different compilers use different amounts of padding. Different machines use various floating point models and may use a different number of bits per byte. In addition, there is always the issue of endianness.
Non-Compliant Code Example
The following non-compliant code reads data from a file stream into a data structure.
struct myData { char c; float f; }; /* ... */ FILE *file; struct myData data; /* initialize file */ if (fread(&data, sizeof(struct myData), 1, file) < sizeof(struct myData)) { /* handle error */ }
However, the code makes assumptions about the layout of myData
, which may be represented differently on a different platform.
Compliant Solution
The best solution is to use either a text representation or a special library that will ensure the integrity of data.
struct myData { char c; float f; }; /* ... */ FILE *file; struct myData data; /* initialize file */ if (fscanf(file, "%c %f\n", &data.c, &data.f) != 2) { /* handle error */ }
Risk Assessment
Reading binary data that has a different format than expected may result in unintended program behavior.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO09-A |
medium |
probable |
high |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Summit 95]], 20.5 on C-FAQ
FIO08-A. Take care when calling remove() on an open file 09. Input Output (FIO) FIO10-A. Take care when using the rename() function