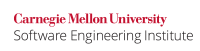
An application programming interface (API) specifies how a function is intended to be called. Calling a function with incorrect arguments can result in unexpected or unintended program behavior. Functions that are appropriately declared (see DCL07-C. Include the appropriate type information in function declarators) will typically fail compilation if they are supplied with the wrong number or types of arguments. However, there are cases where supplying the incorrect arguments to a function will at best generate compiler warnings. These warnings should be resolved (see MSC00-C. Compile cleanly at high warning levels) but do not prevent program compilation.
Noncompliant Code Example (Function Pointers)
In this example, the function pointer fp
is used to refer to the function strchr()
. However, fp
is declared without a function prototype. As a result, there is no type checking performed on the call to fp(12,2);
.
#include <stdio.h> #include <string.h> char *(*fp) (); int main(void) { char *c; fp = strchr; c = fp(12, 2); printf("%s\n", c); }
Compliant Solution (Function Pointers)
Declaring fp
with a function prototype corrects this example.
#include <string.h> char *(*fp) (const char *, int); int main(void) { char *c; fp = strchr; c = fp("Hello",'H'); printf("%s\n", c); }
Noncompliant Code Example (Variadic Functions)
The POSIX function open()
[[Open Group 04]] is a variadic function with the following prototype:
int open(const char *path, int oflag, ... );
The open()
function accepts a third argument to determine a newly created file's access mode. If open()
is used to create a new file and the third argument is omitted, the file may be created with unintended access permissions (see FIO06-C. Create files with appropriate access permissions).
In this noncompliant code example from a vulnerability in the useradd()
function of the shadow-utils
package CVE-2006-1174 , the third argument to open()
has been accidentally omitted.
fd = open(ms, O_CREAT|O_EXCL|O_WRONLY|O_TRUNC);
Compliant Solution (Variadic Functions)
To correct this example, a third argument is specified in the call to open()
.
/* ... */ fd = open(ms, O_CREAT|O_EXCL|O_WRONLY|O_TRUNC, file_access_permissions); if (fd == -1){ /* Handle Error */ } /* ... */
Risk Assessment
Calling a function with incorrect arguments can result in unexpected or unintended program behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP37-C |
medium |
probable |
high |
P4 |
L3 |
Automated Detection
Compass/ROSE could detect the violations in these examples when it can determine which function a function pointer points to at compile time. Then it can match the function's arguments with the expected arguments. If a pointer points to a function not determinable at compile time, ROSE probably won't be able to validate its parameters either.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[CVE]] CVE-2006-1174
[[ISO/IEC 9899:1999]] Forward and Section 6.9.1, "Function definitions"
[[ISO/IEC PDTR 24772]] "OTR Subprogram Signature Mismatch"
[[MISRA 04]] Rule 16.6
[[Spinellis 06]] Section 2.6.1, "Incorrect Routine or Arguments"
EXP36-C. Do not convert pointers into more strictly aligned pointer types 03. Expressions (EXP) EXP38-C. Do not call offsetof() on bit-field members or invalid types