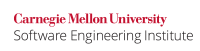
Calling a random number generator (RNG) that is not seeded will result in generating the same sequence of random numbers in different runs of the program.
Suppose there is code that calls an RNG function 10 times to produce a sequence of 10 random numbers. Suppose, also, that this RNG is not seeded. Running the code the first time will produce the sequence S = <r1, r2, r3, r4, r5, r6, r7, r8, r9, r10>. Running the code again a second time will produce the exact same sequence S. Generally, any subsequent runs of the code will generate the same sequence S.
As a result, after the first run of the RNG, an attacker will know the sequence of random numbers that will be generated in future runs. Knowing the sequence of random numbers that will be generated beforehand can lead to many vulnerabilities, especially when security protocols are concerned.
As a solution, you should always ensure that your RNG is properly seeded. Seeding an RNG means that it will generate different sequences of random numbers at any call.
Rule MSC30-CPP. Do not use the rand() function for generating pseudorandom numbers addresses RNGs from a different perspective, i.e., the time until the first collision occurs. In other words, during a single run of an RNG, the time interval after which the RNG generates the same random numbers. Rule MSC30-CPP deprecates the rand()
function because it generates numbers which have a comparatively short cycle. The same rule proposes the use of the random()
function for POSIX and the CryptGenRandom()
function for Windows.
The current rule (MSC32-CPP) examines these three RNGs in terms of seeding. Noncompliant code examples correspond to the use of an RNG without a seed, while compliant solutions correspond to the same RNG being properly seeded. Rule MSC32-CPP addresses all three RNGs mentioned in rule MSC30-CPP for completeness. Rule MSC32-CPP complies to MSC30-CPP and does not recommend the use of the rand()
function. Nevertheless, if it is unavoidable to use rand()
, it should at least be properly seeded.
Noncompliant Code Example
This noncompliant code example generates a sequence of 10 pseudorandom numbers using the rand()
function. When rand()
is not seeded, it uses 1 as a default seed. No matter how many times this code is executed, it always produces the same sequence.
int i=0; for (i=0; i<10; i++) { cout<<rand()<<", "; /* Always generates the same sequence */ } output: 1st run: 41, 18467, 6334, 26500, 19169, 15724, 11478, 29358, 26962, 24464, 2nd run: 41, 18467, 6334, 26500, 19169, 15724, 11478, 29358, 26962, 24464, ... nth run: 41, 18467, 6334, 26500, 19169, 15724, 11478, 29358, 26962, 24464,
Compliant Solution (C Standard)
Use srand()
before rand()
to seed the random sequence generated by rand()
. The code produces different random number sequences at different calls.
srand(time(NULL)); /* Create seed based on current time */ int i=0; for (i=0; i<10; i++) { cout<<rand()<<", "; /* Generates different sequences at different runs */ } output: 1st run: 25121, 15571, 29839, 2454, 6844, 10186, 27534, 6693, 12456, 5756, 2nd run: 25134, 25796, 2992, 403, 15334, 25893, 7216, 27752, 12966, 13931, 3rd run: 25503, 27950, 22795, 32582, 1233, 10862, 31243, 24650, 11000, 7328, ...
Noncompliant Code Example
This noncompliant code example generates a sequence of 10 pseudorandom numbers using the random()
function. When random()
is not seeded, it behaves like rand()
and thus produces the same sequence of random numbers at different calls.
int i=0; for (i=0; i<10; i++) { cout<<random()<<", "; /* Always generates the same sequence */ } output: 1st run: 1804289383, 846930886, 1681692777, 1714636915, 1957747793, 424238335, 719885386, 1649760492, 596516649, 1189641421, 2nd run: 1804289383, 846930886, 1681692777, 1714636915, 1957747793, 424238335, 719885386, 1649760492, 596516649, 1189641421, ... nth run: 1804289383, 846930886, 1681692777, 1714636915, 1957747793, 424238335, 719885386, 1649760492, 596516649, 1189641421,
Compliant Solution (POSIX)
Use srandom()
before random()
to seed the random sequence generated by random()
. The code produces different random number sequences at different calls.
srandom(time(NULL)); /* Create seed based on current time counted as seconds from 01/01/1970 */ int i=0; for (i=0; i<10; i++) { cout<<random()<<", "; /* Generates different sequences at different runs */ } output: 1st run: 198682410, 2076262355, 910374899, 428635843, 2084827500, 1558698420, 4459146, 733695321, 2044378618, 1649046624, 2nd run: 1127071427, 252907983, 1358798372, 2101446505, 1514711759, 229790273, 954268511, 1116446419, 368192457, 1297948050, 3rd run: 2052868434, 1645663878, 731874735, 1624006793, 938447420, 1046134947, 1901136083, 418123888, 836428296, 2017467418, ...
In the previous examples, seeding in rand()
and random()
is done using the time()
function, which returns the current time calculated as the number of seconds that have past since 01/01/1970. Depending on the application and the desirable level of security, a programmer may choose alternative ways to seed RNGs. In general, hardware is more capable of generating real random numbers (for example, generate a sequence of bits by sampling the thermal noise of a diode and use this as a seed).
Compliant Solution (Windows)
The CryptGenRandom()
does not run the risk of not being properly seeded. The reason for that is that its arguments serve as seeders. From the Microsoft Developer Network CryptGenRandom()
reference [MSDN]:
The CryptGenRandom function fills a buffer with cryptographically random bytes.
Syntax
BOOL WINAPI CryptGenRandom(
__in HCRYPTPROV hProv,
__in DWORD dwLen,
__inout BYTE *pbBuffer
);Parameters
hProv [in]
Handle of acryptographic service provider(CSP) created by a call toCryptAcquireContext.
dwLen [in]
Number of bytes of random data to be generated.
pbBuffer [in, out]
Buffer to receive the returned data. This buffer must be at leastdwLenbytes in length.
Optionally, the application can fill this buffer with data to use as an auxiliary random seed.
HCRYPTPROV hCryptProv; union /* union stores the random number generated by CryptGenRandom() */ { BYTE bs[sizeof(long int)]; long int li; } rand_buf; if(CryptAcquireContext(&hCryptProv, NULL, NULL, PROV_RSA_FULL, 0)) /* An example of instantiating the CSP */ { cout<<"CryptAcquireContext succeeded."<<endl; } else { cerr<<"Error during CryptAcquireContext!"<<endl; } for(int i=0;i<10;i++) { if (!CryptGenRandom(hCryptProv, sizeof(rand_buf), (BYTE*) &rand_buf)) { cerr<<"Error during CryptGenRandom"<<endl; } else { cout<<rand_buf.li<<", "; } } output: 1st run: -1597837311, 906130682, -1308031886, 1048837407, -931041900, -658114613, -1709220953, -1019697289, 1802206541, 406505841, 2nd run: 885904119, -687379556, -1782296854, 1443701916, -624291047, 2049692692, -990451563, -142307804, 1257079211, 897185104, 3rd run: 190598304, -1537409464, 1594174739, -424401916, -1975153474, 826912927, 1705549595, -1515331215, 474951399, 1982500583, ...
Risk Assessment
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC32-CPP |
medium |
likely |
low |
P18 |
L1 |
Automated Detection
Compass/ROSE can detect violations of this rule.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This recommendation appears in the C Secure Coding Standard as MSC32-C. Ensure your random number generator is properly seeded.
Bibliography
[C++ Reference] Standard C Library
[MITRE 07] CWE ID 327 , "Use of a Broken or Risky Cryptographic Algorithm," CWE ID 330, "Use of Insufficiently Random Values"
[MSDN] "CryptGenRandom Function"
049. Miscellaneous (MSC) MSC33-CPP. Obey the One Definition Rule