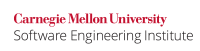
Reclaiming resources when exceptions are thrown is important. An exception being thrown may result in cleanup code being bypassed or an object being left in a partially initialized state. It is preferable that resources be reclaimed automatically, using the RAII design pattern [Stroustrup 2001], when objects go out of scope. This technique avoids the need to write complex cleanup code when allocating resources.
However, constructors do not offer the same protection. Because a constructor is involved in allocating resources, it does not automatically free any resources it allocates if it terminates prematurely. The C++ Standard, [except.ctor], paragraph 2 [ISO/IEC 14882-2014], states:
An object of any storage duration whose initialization or destruction is terminated by an exception will have destructors executed for all of its fully constructed subobjects (excluding the variant members of a union-like class), that is, for subobjects for which the principal constructor (12.6.2) has completed execution and the destructor has not yet begun execution. Similarly, if the non-delegating constructor for an object has completed execution and a delegating constructor for that object exits with an exception, the object’s destructor will be invoked. If the object was allocated in a new-expression, the matching deallocation function (3.7.4.2, 5.3.4, 12.5), if any, is called to free the storage occupied by the object.
It is generally recommended that constructors that cannot complete their job should throw exceptions rather than exit normally and leave their object in an incomplete state [Cline 2009].
Resources must not be leaked as a result of throwing an exception, including during the construction of an object.
Noncompliant Code Example
In this noncompliant code example, pst
is not properly released when processItem
throws an exception, causing a resource leak:
#include <new> struct SomeType { void processItem() noexcept(false); }; void f() { SomeType *pst = new (std::nothrow) SomeType(); if (!pst) { // Handle error return; } try { pst->processItem(); } catch (...) { // Handle error throw; } delete pst; }
Compliant Solution (delete
)
In this compliant solution, the exception handler frees pst
by calling delete
:
#include <new> struct SomeType { void processItem() noexcept(false); }; void f() { SomeType *pst = new (std::nothrow) SomeType(); if (!pst) { // Handle error return; } try { pst->processItem(); } catch (...) { // Handle error delete pst; throw; } delete pst; }
This compliant solution complies with MEM51-CPP. Properly deallocate dynamically allocated resources. However, although handling resource cleanup in catch
clauses works, it can have some disadvantages:
- Each distinct cleanup requires its own
try
andcatch
blocks. - The cleanup operation must not throw any exceptions.
Compliant Solution (RAII Design Pattern)
A better approach is to employ RAII. This pattern forces every object to clean up after itself in the face of abnormal behavior, preventing the programmer from having to do so. Another benefit of this approach is that it does not require statements to handle resource allocation errors, in conformance with MEM52-CPP. Detect and handle memory allocation errors.
struct SomeType { void processItem() noexcept(false); }; void f() { SomeType st; try { st.processItem(); } catch (...) { // Handle error throw; } }
Noncompliant Code Example
In this noncompliant code example, the C::C()
constructor might fail to allocate memory for a
, might fail to allocate memory for b
, or might throw an exception in the init()
method. If init()
throws an exception, neither a
nor b
will be released. Likewise, if the allocation for b
fails, a
will not be released.
#include <new> struct A {/* ... */}; struct B {/* ... */}; class C { A *a; B *b; protected: void init() noexcept(false); public: C() : a(new A()), b(new B()) { init(); } };
Compliant Solution (try/catch
)
This compliant solution mitigates the potential failures by releasing a
and b
if an exception is thrown during their allocation or during init()
:
#include <new> struct A {/* ... */}; struct B {/* ... */}; class C { A *a; B *b; protected: void init() noexcept(false); public: C() : a(nullptr), b(nullptr) { try { a = new A(); b = new B(); init(); } catch (...) { delete a; delete b; throw; } } };
Compliant Solution (std::unique_ptr)
This compliant solution uses std::unique_ptr
to create objects that clean up after themselves should anything go wrong in the C::C()
constructor. See VOID MEM00-CPP. Don't use auto_ptr where copy semantics might be expected for more information on std::unique_ptr
.
#include <memory> struct A {/* ... */}; struct B {/* ... */}; class C { std::unique_ptr<A> a; std::unique_ptr<B> b; protected: void init() noexcept(false); public: C() : a(new A()), b(new B()) { init(); } };
Risk Assessment
Memory and other resource leaks will eventually cause a program to crash. If an attacker can provoke repeated resource leaks by forcing an exception to be thrown through the submission of suitably crafted data, then the attacker can mount a denial-of-service attack.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ERR57-CPP | Low | Probable | High | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
|
|
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
SEI CERT C++ Coding Standard | MEM52-CPP. Detect and handle memory allocation errors MEM51-CPP. Properly deallocate dynamically allocated resources |
Bibliography
[ISO/IEC 14882-2014] | 15.2, "Constructors and Destructors" |
[Meyers 96] | Item 9: "Use destructors to prevent resource leaks" |
[Stroustrup 2001] | "Exception-Safe Implementation Techniques" |
[Cline 2009] | 17.2, "I'm still not convinced: A 4-line code snippet shows that return-codes aren't any worse than exceptions; |