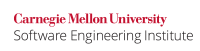
Avoid the use of in-band error indicators. These are much less common in Java than in some other programming languages, but they are used in the read(byte[] b, int off, int len)
and read(char[] cbuf, int off, int len)
family of methods in java.io
.
Noncompliant Code Example
This noncompliant code example attempts to read into an array of characters and the add an extra character into the buffer immediately after the characters read.
static final int MAX = 21; static final int MAX_READ = MAX - 1; static final char TERMINATOR = '\\'; int read; char [] chBuff = new char [MAX]; BufferedReader buffRdr; // set up buffRdr read = buffRdr.read(chBuff, 0, MAX_READ); chBuff[read] = TERMINATOR;
However, if the input buffer is initially at end-of-file then the read
method will return -1 and the attempt to place the termintor character will throw an ArrayIndexOutOfBoundsException
.
Compliant Solution
This compliant solution defines a readSafe
method that throws an exception if end-of-file is detected.
class SafeBufferedReader extends BufferedReader { SafeBufferedReader(Reader in) { super(in); } public int readSafe(char[] cbuf, int off, int len) throws EOFException { int read = read(cbuf, off, len); if (read == -1) { throw new EOFException(); } else { return read; } } } // ... SafeBufferedReader safeBuffRdr; try { read = safeBuffRdr.readSafe(chBuff, 0, MAX_READ); chBuff[read] = TERMINATOR; } catch (EOFException eof) { chBuff[0] = TERMINATOR; }
Risk Assessment
Using in-band error indicators may result in programmers failing to check status codes or using incorrect return values.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ERR53-J |
low |
unlikely |
high |
P1 |
L3 |
Automated Detection
Given the comparatively rare occurrence of in-band error indicators in Java, it may be possible to compile a list of all methods that use them and automatically detect their use. However, detecting the safe use of in-band error indicators is not feasible.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="5bb935e6-b202-46f7-a7d2-32201e2620d7"><ac:plain-text-body><![CDATA[ |
[[API 2006 |
AA. References#API 06]] |
[Class Reader |
http://download.oracle.com/javase/6/docs/api/java/io/Reader.html] |
]]></ac:plain-text-body></ac:structured-macro> |