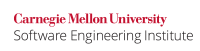
Java's Object cloning mechanism can allow an attacker to manufacture new instances of a class that has been defined without executing its constructor. If a class is not cloneable, the attacker can define a subclass and make the subclass implement the java.lang.Cloneable interface. This lets the attacker create new instances of the class. The new instances of the class are made by copying the memory images of existing objects. Although this is sometimes an acceptable way of making a new object, it often is not.
Noncompliant Code Example
Consider the following class definition. Unless someone knows the secret password, objects cannot be created because the constructor for the class checks for the password stored in some password file.
class MyPrivacy { //define class member fields //... public MyPrivacy(String passwd) { String actualPass; FileReader fr = new FileReader("Passfile.txt"); BufferedReader br = new BufferedReader(fr); actualPass = br.readLine(); if(actualPass.equals(passwd)){ // return normally } else{ // exit the program, print an authentication error // preventing the class object from being created } } public void use(){ // } //... }
The attacker can create a new instance of MyPrivacy class by using a cloneable subclass and bypass the constructor. Bypassing the constructor leads to bypassing the password check done in the constructor.
class Test extends MyPrivacy implements Cloneable{ public static void somefunction(MyPrivacy obj) { try { Test t = (Test)obj.clone() }catch(Exception e) { System.out.println("not cloneable"); } if (t != null) t.use(); // Another object instantiated without knowing the password..... } }
Compliant Solution
Classes should be made noncloneable to prevent this from occurring. The following method may be implemented to achieve this.
class MyPrivacy { //define class member fields //... public MyPrivacy(String passwd) { String actualPass; FileReader fr = new FileReader("Passfile.txt"); BufferedReader br = new BufferedReader(fr); actualPass = br.readLine(); if(actualPass.equals(passwd)){ // return normally } else{ // exit the program, print an authentication error // preventing the class object from being created } } public void use(){ // } //... public final void clone() throws java.lang.CloneNotSupportedException{ throw new java.lang.CloneNotSupportedException(); } }
Compliant Solution
One can also make a class nonsubclassable. This can be achieved by finalizing a class.
 final class MyPrivacy { // Rest of the definition remains the same }
If it is absolutely required to make the class cloneable, even then protective measures can be taken.
- If the clone method is being overriden, make it final.
- If the class is reliant on a nonfinal clone method of one of the superclasses, then define the following:
public final void clone() throws java.lang.CloneNotSupportedException { super.clone(); }
Risk Assessment
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC05-J |
Medium |
Probably |
medium |
8 |
L2 |
References
http://www.javaworld.com/javaworld/jw-12-1998/jw-12-securityrules.html?page=4
http://www.dwheeler.com/secure-programs/Secure-Programs-HOWTO/java.html
MSC04-J. Be aware of JVM Monitoring and Managing 11. Miscellaneous (MSC) MSC30-J. Generate truly random numbers