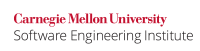
Java is considered to be a safer language than C or C++. The following excerpt is from the introduction of secure coding guidelines from SUN SUN secure coding :
"The (java) language is type-safe, and the runtime provides automatic memory management and range-checking on arrays. These features also make Java programs immune to the stack-smashing and buffer overflow attacks possible in the C and C++ programming languages, and that have been described as the single most pernicious problem in computer security today"
While this statement is in fact true, the arithmetic operations in the Java platform require the same caution as in C\C++. Integer operations can result in overflow or underflow since Java does not provide any indication of these conditions and silently wraps (Java throws only a division by zero exception).
The following excerpt is from the Java Language Specification (Overflow)
"The built-in integer operators do not indicate overflow or underflow in any way. Integer operators can throw a NullPointerException if unboxing conversion of a null reference is required. Other than that, the only integer operators that can throw an exception are the integer divide operator / and the integer remainder operator %, which throw an ArithmeticException if the right-hand operand is zero, and the increment and decrement operators ++ and - which can throw an OutOfMemoryError if boxing conversion is required and there is not sufficient memory available to perform the conversion"
See the following example:
Noncompliant Code Example
In we have the following simple method the result could overflow
public int do_operation(int a,int b) { int temp = a + b; //Could result in overflow //do other processing return temp; }
If the result of the addition, is greater than the maximum value that the int type can store or less than the minimum value that the int type can store, then the variable temp has a wrong result stored. Although, unlike C\C++ the integer overflow is almost impossible to exploit in Java because of the memory properties in this platform (e.g. explicit array bound checking; if temp has a negative value as a result of an overflow and we use it as an array index we get an java.lang.ArrayIndexOutOfBoundsException) the results of our operation are wrong and can lead to undefined or incorrect behavior
All the of the following operators can lead to overflow (same as in C\C++):
Operator |
Overflow |
|
Operator |
Overflow |
|
Operator |
Overflow |
|
Operator |
Overflow |
---|
| + | yes | | -= | yes | | << | yes | | < | no |
yes |
|
*= |
yes |
|
>> |
no |
|
> |
no |
||
yes |
|
/= |
yes |
|
& |
no |
|
>= |
no |
||
/ |
yes |
|
%= |
yes |
|
| |
no |
|
<= |
no |
|
% |
yes |
|
<<= |
yes |
|
^ |
no |
|
== |
no |
|
++ |
yes |
|
>>= |
no |
|
~ |
no |
|
!= |
no |
|
-- |
yes |
|
&= |
no |
|
! |
no |
|
&& |
no |
|
= |
no |
|
|= |
no |
|
un+ |
no |
|
|| |
no |
|
+= |
yes |
|
^= |
no |
|
un- |
yes |
|
?: |
no |
|
Addition
Addition (and all operations) in Java are performed in signed numbers as Java does not support unsigned numbers
Noncompliant Code Example
In this example the addition could result in overflow
public int do_operation(int a,int b) { int temp = a + b; //Could result in overflow //do other processing return temp; }
Compliant Solution (Bounds Checking)
A solution would be to explicitly check the range of each arithmetic operation and throw an ArithmeticException on overflow, otherwise downcast the value to an integer. For arithmetical operations on really big numbers one should always use the BigInteger Class
In this platform according to SUN Java Data Types:
-the integer data type is a 32-bit signed two's complement integer. It has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive).
- the long data type is a 64-bit signed two's complement integer. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive). Use this data type when you need a range of values wider than those provided by int
So since long is guaranteed to be able to hold the result of an int addition, we could assign the result to a long and if the result is in the integer range we simply downcast. All of the tests would be the same as with signed integers in C since Java does not support unsigned numbers
e.g for the previous example
public int do_operation(int a, int b) throws ArithmeticException { long temp = (long)a+(long)b; if(temp >Integer.MAX_VALUE || temp < Integer.MIN_VALUE) throw ArithmeticException; else //Value within range can perform the addition //Do stuff return (int)temp; }
Another example would be of explicit range checking would be:
public int do_operation(int a, int b) throws ArithmeticException { int temp; if(a>0 && b>0 && (a >Integer.MAX_VALUE - b) || a<0 && b<0 && (a < Integer.MIN_VALUE -b)) throw ArithmeticException; else temp = a + b;//Value within range can perform the addition //Do stuff return temp; }
Another compliant approach would be to use the BigInteger class in this example and in the examples of the other operations using a wrapper for test of the overflow:
public bool overflow(int a, int b) { java.math.BigInteger ba = new java.math.BigInteger(String.valueOf(a)); java.math.BigInteger bb = new java.math.BigInteger(String.valueOf(b)); java.math.BigInteger br = ba.add(bb); if(br.compareTo(java.math.BigInteger.valueOf(Integer.MAX_VALUE)) == 1 || br.compareTo(java.math.BigInteger.valueOf(Integer.MIN_VALUE))== -1) return true;//We have overflow //Can proceed return false } public int do_operation(int a, int b) throws ArithmeticException { if overflow(a,b) throw ArithmeticException; else //we are within range safely perform the addition }
By using the BigInteger class there is no chance to overflow (see section on BigInteger class) but the performance is degraded so it should be used only on really large operations
Subtraction
Care must be taken in subtraction operations as well as these can overflow as well.
Noncompliant Code Example
public int do_operation(int a,int b) { int temp = a - b; //Could result in overflow //perform other processing return temp; }
Compliant Code Example
int a,b,result; long temp = (long)a-(long)b; if(long < Integer.MIN_VALUE || long > Integer.MAX_VALUE) throw ArithmeticException; else result = (int) temp;
Multiplication
This noncompliant code example, can result in a signed integer overflow during the multiplication of the signed operands a and b. If this behaviour is unanticipated, the resulting value may lead to undefined behaviour
Noncompliant Code Example
int a,b,result //do stuff result = a*b;//May result in overflow
Compliant Code Example
Since in this platform the size of type long (64 bits) is twice the size of type int (32 bits) we should perform the multiplication in terms of long and if the product is in the integer range we downcast the result to int
int a,b,result; long temp = (long) a\* (long)b; if(temp > Integer.MAX_VALUE || temp < Integer.MIN_VALUE) throw ArithmeticException;//overflow else result = (int) temp;//Value within range, safe to downcast
Division
Although Java throws a java.lang.ArithmeticException: / by zero exception for division by zero, there is the same issue as in C\C++ when dividing the Integer.MIN_VALUE with -1. It produces Integer.MIN_VALUE unexpectedly
(since the result is -(Integer.MIN_VALUE)=Integer.MAX_VALUE +1))
A non-compliant example is:
Noncompliant Code Example
int a,b,result result = a/b;
Compliant Code Example
if(a == Integer.MIN_VALUE && b == -1) throw ArithmeticException;//May be Integer.MIN_VALUE again???? else result = a/b;//safe operation
Division
For modulo operator the only problem is if we take the modulo of Integer.MIN_VALUE with -1. The result is always 0 in JAVA so we are back to the previous rule (for division)
Unary Negation
If we negate Integer.MIN_VALUE we get Integer.MIN_VALUE. So we explicitely check the range
if(a == Integer.MIN_VALUE) throw ArithmeticException; else result = --a;
SHIFTING
The shift in java is quite different than in C\C++.
1) The right shift in java is an arithmetic shift while in C\C++ is implementation defined (logical or arithmetic)
2) In C\C++ if the value being left shifted is negative or the right hand operator of the shift operation is negative or greater than or equal to the width of the promoted left operand we have umdefined behaviour. This does not apply in Java since for the case of integer type it is masked with 0x1F and as a result we can always have a value that is modulo 31. When the value to be shifted (left-operand) is a long, only the last 6 bits of the right-hand operand are used to perform the shift. The actual size of the shift is the value of the right-hand operand masked by 63 (0x3D) Java Language Specification(§15.19 )
ie the shift distance is always between 0 and 63 (if shift value is greater than 64 shift is 64%value)
35 00000000 00000000 00000000 00100011
31 -> 0x1f 00000000 00000000 00000000 00011111
& -----------------------------------
Shift value 00000000 00000000 00000000 00000011 -> 3
So according to JLS
"At run time, shift operations are performed on the two's complement integer representation of the value of the left operand. The value of n<<s is nleft-shifted s bit positions; this is equivalent (even if overflow occurs) to multiplication by two to the power s.The value of n>>s is n right-shifted s bit positions with sign-extension. The resulting value is
?n/2s?. For nonnegative values of n, this is equivalent to truncating integer division, as computed by the integer division operator /, by two to the power s."
3) There is a new operator in Java <<< that performs unsigned right shift
Example:
int val = 2 <<-29; int val = 2 << 35; These both print 16 because they are transformed to 2<<3
Although we can not have undefined behaviour in Java we still have to ensure that we get the correct results. So we should
explicitly check for ranges
if(shift_value > 31 or shift_value <0)if(shift_value > 31 or shift_value <0) throw ArithmeticException; else int val = 2 << shift_value; throw ArithmeticException; else int val = 2 << shift_value;
Unsigned Right shifting >>>
It is identical to the right-shift operator if the shifted value is positive. If it is negative the sign value can
change because the left-operand high-order bit is not retained and the sign value can change; Excerpt
from JLS:
"if n is negative, the result is equal to that of the expression (n>>s)(2<<~s) if the type of the left-hand operand is int, and to the result of the expression (n>>s)(2L<<~s) if the type of the left-hand operand is long. The added term (2<<~s) or (2L<<~s) cancels out the propagated sign bit. (Note that, because of the implicit masking of the right-hand operand of a shift operator, ~s as a shift distance is equivalent to 31-s when shifting an int value and to 63-s when shifting a longvalue.)"
For example: -32 >>> 2 = (-32 >> 2 ) + ( 2 << ~2 ) = 1073741816
Operations Requiring Really Long Numbers
For these operations the BigInteger class should be used. According to SUN BigInteger Class:
"Semantics of arithmetic operations exactly mimic those of Java's integer arithmetic operators, as defined in The Java Language Specification. For example, division by zero throws an ArithmeticException
, and division of a negative by a positive yields a negative (or zero) remainder. All of the details in the Spec concerning overflow are ignored, as BigIntegers are made as large as necessary to accommodate the results of an operation."
So operations using BigInteger class are guaranteed not to overflow regardless of the size of the result.
For instance operations on long are operations on 64 bits. For example addition:
Compliant Code Example
java.math.BigInteger big_long_max = new java.math.BigInteger(String.valueOf(Long.MAX_VALUE)); System.out.println("big_long="+big_long_max); big_long_max = big_long_max.add(java.math.BigInteger.valueOf(1));//same as ++big_long_max System.out.println("big_long="+big_long_max); These print big_long=9223372036854775807 big_long=9223372036854775808//exceeds the maximum range of long, no problem java.math.BigInteger big_long_min = new java.math.BigInteger(String.valueOf(Long.MIN_VALUE)); System.out.println("big_long_min="+big_long_min); big_long_min = big_long_min.subtract(java.math.BigInteger.valueOf(1));//same as --big_long_min System.out.println("big_long_min="+big_long_min);//goes bellow minimum range of long, no problem These print: big_long_min=-9223372036854775808 big_long_min=-9223372036854775809 if(big_long < Long.MAX_VALUE && big_long > Long.MIN_VALUE)//value within range can go to the primitive type long value = big_log.longValue();//get primitive type else //Perform error handling. We can not downcast since the value can not be represented as a long
We can always go back to the primitive types if the BigInteger of course can be represented by the type
In the example if big_long is within long range (big_long < Long.MAX_VALUE && big_long > Long.MIN_VALUE) we can use the BigInteger method longValue() to get the long value and assign it to a variable of type long