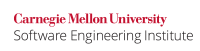
"An inner class is a nested class that is not explicitly or implicitly declared static." [[JLS 05]]. Serialization of inner classes (including local and anonymous classes) is error prone. According to the Serialization Specification [[Sun 06]]:
- Because inner classes declared in non-static contexts contain implicit non-transient references to enclosing class instances, serializing such an inner class instance results in serialization of its associated outer class instance.
- Synthetic fields generated by
javac
(or other JavaTM
compilers) to implement inner classes are implementation dependent and may vary between compilers; differences in such fields can disrupt compatibility as well as result in conflicting default serialVersionUID values. The names assigned to local and anonymous inner classes are also implementation dependent and may differ between compilers.
- Because inner classes cannot declare
static
members other than compile-time constant fields, they cannot use theserialPersistentFields
mechanism to designate serializable fields.
Finally, because inner classes associated with outer instances do not have zero-argument constructors (constructors of such inner classes implicitly accept the enclosing instance as a prepended parameter), they cannot implement
Externalizable
. [TheExternalizable
interface requires the implementing object to manually save and restore its state using thewriteExternal()
andreadExternal()
methods.]
None of these issues, however, apply to static
member classes.
Noncompliant Code Example
In this noncompliant code example, the fields contained within the outer class are also serialized when the inner class is serialized.
public class OuterSer implements Serializable { private int ssn; class InnerSer implements Serializable { protected String name; //... } }
Compliant Solution
This compliant solution recommends against implementing the Serializable
interface in the InnerSer
class.
public class OuterSer implements Serializable { private int ssn; class InnerSer { protected String name; //... } }
Compliant Solution
It is also allowable to declare the inner class as static
to prevent its serialization.
public class OuterSer implements Serializable { private int ssn; static class InnerSer { protected String name; //... } }
Risk Assessment
Attempts to serialize inner classes can cause instances of the outer class to be serialized and also discourage platform independence.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SER33- J |
medium |
likely |
low |
P18 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
[[JLS 05]] Section 8.1.3, Inner Classes and Enclosing Instances
[[Sun 06]] "Serialization specification:
[[Bloch 08]] Item 74: "Implement serialization judiciously"
SER32-J. Do not allow serialization and deserialization to bypass the Security Manager 12. Serialization (SER) SER34-J. Make defensive copies of private mutable components