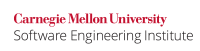
Because an exception is caught by its type, it is better to define exceptions for specific purposes than to use the general exception types for multiple purposes. Throwing the general exception types makes code hard to understand and maintain and defeats much of the advantage of the Java exception-handling mechanism.
Noncompliant Code Example
This noncompliant code example attempts to distinguish between different exceptional behaviors by looking at the exception's message:
try { doSomething(); } catch (Throwable e) { String msg = e.getMessage(); switch (msg) { case "stack underflow": // Handle error break; case "connection timeout": // Handle error break; case "security violation": // Handle error break; default throw e; } }
If doSomething
throws an exception whose type is a subclass of Exception
with the message "stack underflow," the appropriate action will be taken in the exception handler. However, any change to the literals involved will break the code. For example, if a maintainer were to edit the throw expression to read throw new Exception("Stack Underflow");
, the exception would be rethrown by the code of the noncompliant code example rather than handled. Finally, exceptions may be thrown without a message.
This noncompliant code example falls under ERR08-EX0 of rule ERR08-J. Do not catch NullPointerException or any of its ancestors.
Compliant Solution
It is better to use existing specific exception types or to define new special-purpose exception types:
public class StackUnderflowException extends Exception { StackUnderflowException () { super(); } StackUnderflowException (String msg) { super(msg); } } // ... try { doSomething(); } catch (StackUnderflowException sue) { // Handle error } catch (TimeoutException te) { // Handle error } catch (SecurityException se) { // Handle error }
Applicability
Exceptions are used to handle exceptional conditions. If an exception is not caught, the program will be terminated. An exception that is incorrectly caught or is caught at the wrong level of recovery will often cause incorrect behavior.
Bibliography