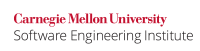
The Java compiler type-checks the arguments to each variable arity (varargs) method to ensure that the arguments are of the same type or object reference. However, the compile-time checking is ineffective when Object
or generic parameter types are used [Bloch 2008]. The presence of initial parameters of specific types is irrelevant; the compiler will remain unable to check Object
or generic variable arity parameter types. Enable strong compile-time type checking of variable arity methods by using the most specific type possible for the type of the method parameter.
Noncompliant Code Example (Object
)
This noncompliant code example declares a variable arity method using Object
. It accepts an arbitrary mix of parameters of any object type. Legitimate uses of such declarations are rare (but see below in the "Applicability" section).
ReturnType method(Object... args) { }
Noncompliant Code Example (Generic Type)
This noncompliant code example declares a variable arity method using a generic type parameter. It accepts a variable number of parameters that are all of the same object type. Again, legitimate uses of such declarations are rare.
<T> ReturnType method(T... args) { }
Compliant Solution
Be as specific as possible when declaring parameter types; avoid Object
and imprecise generic types in variable arity methods.
ReturnType method(SpecificObjectType... args) { }
Retrofitting old methods containing final array parameters with generically typed variable arity parameters is not always a good idea. For example, given a method that does not accept an argument of a particular type, it could be possible to override the compile-time checking — through the use of generic variable arity parameters — so that the method would compile cleanly rather than correctly, causing a run-time error [Bloch 2008].
Also, note that autoboxing prevents strong compile-time type checking of primitive types and their corresponding wrapper classes.
Applicability
Injudicious use of variable arity parameter types prevents strong compile-time type checking, creates ambiguity, and diminishes code readability.
Variable arity signatures using Object
and imprecise generic types are acceptable when the body of the method lacks both casts and autoboxing, and also compiles without error. Consider the following example, which operates correctly for all object types and type-checks successfully.
Collection<T> assembleCollection(T... args) { Collection<T> result = new HashSet<T>(); // add each argument to the result collection return result; }
In some circumstances, it is necessary to use a variable arity parameter of type Object
. A good example of this is the method java.util.Formatter.format(String format, Object... args)
which can format objects of any type.
Automated detection appears to be straightforward.
Bibliography
Item 42: "Use Varargs Judiciously" | |
"Using the Varargs Language Feature" | |
[Sun 2006] |