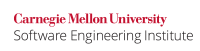
The method java.lang.Object.equals()
by default, is unable to compare composite objects such as cryptographic keys. Most Key
classes do not provide an equals()
implementation that overrides Object's default implementation. In such cases, the components of the composite object must be compared individually to ensure correctness.
Noncompliant Code Example
This noncompliant code example compares two keys using the equals()
method but the keys may compare unequal even if they represent the same key.
private static boolean keysEqual(Key key1, Key key2) { if (key1.equals(key2)) { return true; } }
Compliant Solution
This compliant solution uses the equals()
method as a first test and then compares the encoded version of the keys to facilitate provider-independent behavior. For example, it can be checked if a RSAPrivateKey
and RSAPrivateCrtKey
represent an equivalent private key [Sun 2006].
private static boolean keysEqual(Key key1, Key key2) { if (key1.equals(key2)) { return true; } if (Arrays.equals(key1.getEncoded(), key2.getEncoded())) { return true; } // More code for different types of keys here. // For example, the following code can check if // an RSAPrivateKey and an RSAPrivateCrtKey are equal: if ((key1 instanceof RSAPrivateKey) && (key2 instanceof RSAPrivateKey)) { if ((((RSAKey) key1).getModulus().equals(((RSAKey) key2).getModulus())) && (((RSAPrivateKey) key1).getPrivateExponent().equals( ((RSAPrivateKey) key2).getPrivateExponent()))) { return true; } } return false; }
Risk Assessment
Using Object.equals()
to compare cryptographic keys may not yield accurate results.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC04-J |
high |
unlikely |
low |
P9 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[API 2006]
[Sun 2006] Determining If Two Keys Are Equal (JCA Reference Guide)
MSC03-J. Never hardcode sensitive information 49. Miscellaneous (MSC) MSC05-J. Store passwords using a hash function