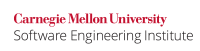
It is tempting to consider primitive operations on primitive data atomic. For instance, you can assume that the code:
x = 3;
is atomic with regard to x. Many people also assume atomicity in operators like the following:
x++;
But this is not an atomic operator; it involves reading the current value of x
, adding one to it, and setting x
to the new, incremented value.
Declaring a shared mutable variable volatile
ensures the visibility of the latest updates on it across other threads but does not guarantee the atomicity of composite operations. For example, the variable increment operation consisting of the sequence read-modify-write is not atomic even when the variable is declared volatile
.
"Sequential consistency and/or freedom from data races still allows errors arising from groups of operations that need to be perceived atomically and are not." [[JLS 05]]. In such cases, the java.util.concurrent
utilities must be used to atomically manipulate a shared variable. If the utilities do not provide the required atomic methods, operations that use the variable should be correctly synchronized.
Note that, as with volatile
, updated values are immediately visible to other threads when either one of these two techniques is used. Synchronization provides a way to safely share object state across multiple threads without the need to reason about reorderings, compiler optimizations and hardware specific behavior.
This rule specifically deals with primitive operators like ++
. For atomicity of other functions, see CON07-J. Do not assume that a grouping of calls to atomic methods is atomic.
The Java Specification also permits reads and writes of 64-bit values to be non-atomic; for more information, see CON25-J. Ensure atomicity when reading and writing 64-bit values.
Noncompliant Code Example (volatile
)
In this noncompliant code example, the volatile
field itemsInInventory
can be accessed by multiple threads. However, when a thread is updating the value of itemsInInventory
, it is possible for other threads to read the original value (that is, the value before the update). This is because the post decrement operator is non-atomic.
private volatile int itemsInInventory = 100; public int removeItem() { if(itemsInInventory > 0) { return itemsInInventory--; // Returns new count of items in inventory } return -1; // Error code }
Compliant Solution (java.util.concurrent.atomic classes)
Volatile variables are unsuitable when more than one load/store operation needs to be atomic. There is an alternative method to perform multiple operations atomically. This compliant solution uses a java.util.concurrent.atomic.AtomicInteger
variable.
public class Sync { private final AtomicInteger itemsInInventory = new AtomicInteger(100); private int removeItem() { for (;;) { int old = itemsInInventory.get(); if (old > 0) { int next = old - 1; if (itemsInInventory.compareAndSet(old, next)) { return next; //returns new count of items in inventory } } else { return -1; // Error code } } } }
According to the Java API [[API 06]], class AtomicInteger
documentation:
[AtomicInteger is] An
int
value that may be updated atomically. AnAtomicInteger
is used in applications such as atomically incremented counters, and cannot be used as a replacement for anInteger
. However, this class does extendNumber
to allow uniform access by tools and utilities that deal with numerically-based classes.
The compareAndSet()
method takes two arguments, the expected value of a variable when the method is invoked and the updated value. This compliant solution uses this method to atomically set the value to the given updated value if and only if the current value equals the expected value. [[API 06]]
Compliant Solution (method synchronization)
This compliant solution uses method synchronization to synchronize access to shared variables. Consequently, access to itemsInInventory
is mutually exclusive and consistent across all object states.
private int itemsInInventory = 100; public synchronized int removeItem() { if(itemsInInventory > 0) { return itemsInInventory--; // Returns new count of items in inventory } return -1; // Error Code }
Synchronization is more expensive than using the optimized java.util.concurrent
utilities and should only be used when the utilities do not contain the facilities (methods) required to carry out the atomic operation. When using explicit synchronization, the programmer must also ensure that two or more threads are not mutually accessible from a different set of two or more threads such that each thread holds a lock while trying to obtain another lock that is held by the other thread [[Lea 00]]. Failure to follow this advice results in deadlocks ([CON12-J. Avoid deadlock by requesting and releasing locks in the same order]).
Compliant Solution (block synchronization)
Constructors and methods can use an alternative representation called block synchronization which synchronizes a block of code rather than a method, as highlighted in this compliant solution.
private int itemsInInventory = 100; public int removeItem() { synchronized(this) { if(itemsInInventory > 0) { return itemsInInventory--; // Returns new count of items in inventory } return -1; // Error code } }
Block synchronization is preferable over method synchronization because it reduces the duration for which the lock is held and also protects against denial of service attacks, though the latter requires synchronizing on a private
lock object instead of the this
reference.
If the synchronized
block was moved inside the if
condition to reduce the performance cost associated with synchronization, the variable itemsInInventory
would be required to be declared as volatile
because the check to determine whether it is greater than 0 relies on the latest value of the variable.
Compliant Solution (ReentrantLock
)
This compliant solution uses a java.util.concurrent.locks.ReentrantLock
to atomically perform the operation.
public class Sync { private int itemsInInventory = 100; private final Lock lock = new ReentrantLock(); public int removeItem() { Boolean myLock = false; try { myLock = lock.tryLock(); if(itemsInInventory > 0) { return itemsInInventory--; } } finally { if (myLock) { lock.unlock(); } } return -1; // Error code } }
Code that uses this lock behaves similar to synchronized code that uses the traditional monitor lock. In addition, it provides several other capabilities, for instance, the tryLock()
method does not block waiting if another thread is already holding the lock. The class java.util.concurrent.locks.ReentrantReadWriteLock
can be used when a thread requires a lock to write information while other threads require the lock to simultaneously read the information.
Risk Assessment
If operations on shared variables are not atomic, unexpected results may be produced. For example, there can be inadvertent information disclosure as one user may be able to receive information about other users.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON01- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class AtomicInteger
[[JLS 05]] Chapter 17, Threads and Locks, section 17.4.5 Happens-before Order, section 17.4.3 Programs and Program Order, section 17.4.8 Executions and Causality Requirements
[[Tutorials 08]] Java Concurrency Tutorial
[[Lea 00]] Sections, 2.2.7 The Java Memory Model, 2.2.5 Deadlock, 2.1.1.1 Objects and locks
[[Bloch 08]] Item 66: Synchronize access to shared mutable data
[[Daconta 03]] Item 31: Instance Variables in Servlets
[[JavaThreads 04]] Section 5.2 Atomic Variables
[[Goetz 06]] 2.3. "Locking"
[[MITRE 09]] CWE ID 667 "Insufficient Locking", CWE ID 413
"Insufficient Resource Locking", CWE ID 366
"Race Condition within a Thread", CWE ID 567
"Unsynchronized Access to Shared Data"
11. Concurrency (CON) 11. Concurrency (CON) CON02-J. Always synchronize on the appropriate object