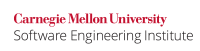
Programmers frequently make errors regarding the precedence rules of operators due to the unintuitive low-precedence levels of &
, |
, ^
, <<
, and >>
. Mistakes regarding precedence rules can be avoided by the suitable use of parentheses. Defensive use of parentheses, if not taken to excess, also improves code readability.
The Java Tutorials defines the precedence of operation by the order of the subclauses.
This recommendation is similar to EXP30-J, however it applies to more than expressions containing side effects.
Noncompliant Code Example
The intent of the expression in this noncompliant code example is to add the variable OFFSET
with the result of the bitwise
AND
between x
and MASK
.
public static final int MASK = 1337; public static final int OFFSET = -1337; public static int computeCode(int x) { return x & MASK + OFFSET; }
Due to operator precedence rules, the expression is parsed as:
x & (MASK + OFFSET)
This gets evaluated as shown below, resulting in the value 0.
x & (1337 - 1337)
Compliant Solution
In this compliant solution, parentheses are used to ensure that the expression evaluates as expected.
public static final int MASK = 1337; public static final int OFFSET = -1337; public static int computeCode(int x) { return (x & MASK) + OFFSET; }
Exceptions
EXP00-EX1: Mathematical expressions that follow algebraic order do not require parentheses. For instance, consider the expression:
x + y * z
By mathematical convention, multiplication is performed before addition. Consequently, parentheses may prove to be redundant in this case.
x + (y * z)
Risk Assessment
Mistakes regarding precedence rules may cause an expression to be evaluated in an unintended way. This can lead to unexpected and abnormal program behavior.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP09-J |
low |
probable |
medium |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Coding Standard as EXP00-C. Use parentheses for precedence of operation.
This rule appears in the C++ Secure Coding Standard as EXP00-CPP. Use parentheses for precedence of operation.
References
[[Tutorials 08]] Expressions, Statements, and Blocks