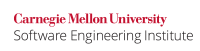
The Java Singleton pattern is a design pattern that governs the instantiation process. According to this design pattern, there can only be one instance of your class per JVM at any time.
The most usual implementation of a Singleton in Java is done by having a single instance of the class as a static field.
You can create that instance using lazy initialization, which means that the instance is not created when the class loads, but when it is first used.
Noncompliant Code Example
When the getter method is called by two threads or classes simultaneously can lead in multiple instances of the singleton class if you neglect to use synchronization, which is a common mistake that happens with that implementation.
public class MySingleton { private static MySingleton _instance; private MySingleton() { // construct object . . . // private constructor prevents instantiation by outside callers } // lazy initialization //error, no synchronization on method public static MySingleton getInstance() { if (_instance==null) { _instance = new MySingleton(); } return _instance; } // Remainder of class definition . . . }
Noncompliant Code Example
Multiple instances can be created even if you add a synchronized(this) block to the constructor call.
// Also an error, synchronization does not prevent // two calls of constructor. public static MySingleton getInstance() { if (_instance==null) { synchronized (MySingleton.class) { _instance = new MySingleton(); } } return _instance; }
Compliant Solution
To avoid that, make the getInstance() a synchronized method.
public class MySingleton { private static MySingleton _instance; private MySingleton() { // construct object . . . // private constructor prevents instantiation by outside callers } // lazy initialization public static synchronized MySingleton getInstance() { if (_instance==null) { _instance = new MySingleton(); } return _instance; } // Remainder of class definition . . . }
By applying a static modifier to the getInstance()method which returns the Singleton, allows the method to be accessed subsequently (after the initial call) without creating a new object.
Noncompliant Code Example
Anther solution for Singletons to be thread-safe is the double-checked locking. It is not guaranteed to work because compiler optimizations can make the assignment of the new Singleton object before all its fields are initialized.
// double-checked locking public static MySingleton getInstance() { if (_instance==null) { synchronized (MySingleton.class) { if (_instance==null) { _instance = new MySingleton(); } } } }
Compliant Solution
It is still possible to create a copy of the Singleton object by cloning it using the Object's clone method, which violates the Singleton Design Pattern's objective. To prevent that you need to override the Object's clone method, which throws a CloneNotSupportedException exception.
public class MySingleton { private static MySingleton _instance; private MySingleton() { // construct object . . // private constructor prevents instantiation by outside callers } // lazy initialization public static synchronized MySingleton getInstance() { if (_instance==null) { _instance = new MySingleton(); } return _instance; } public Object clone() throws CloneNotSupportedException { throw new CloneNotSupportedException(); } // Remainder of class definition . . . }
By applying a static modifier to the getInstance()method which returns the Singleton, allows the method to be accessed subsequently without creating a new object.
Risk Assessment
To guarantee the liveness of a system, the method notifyAll()
should be called rather than notify()
.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON32-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the Java Secure Coding Standard as MSC05-J. Make your classes non Cloneable unless required
References
TODO
[[JLS 05]] Chapter 17, Threads and Locks
CON31-J. Always invoke the wait() method inside a loop 08. Concurrency (CON) 09. Methods (MET)