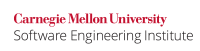
Propagating the content of exceptions without performing explicit filtering is often associated with information leakage. An attacker may craft input parameters such that underlying structures and mechanisms may get exposed inadvertently. Information leakage can result from both the exception message text and the type of exception. For example, with FileNotFoundException
, the message reveals the file system layout while the type conveys the absence of the file.
Noncompliant Code Example
This example demonstrates code that accepts a file name as an input argument on which operations are to be performed. An attacker can gain insights on the underlying file system structure by repeatedly passing different paths to fictitious files. When a file is not found, the FileInputStream
constructor throws a FileNotFoundException
. Other risks such as revelation of the user's home directory and as a result the user name also manifest themselves.
import java.io.FileInputStream; import java.io.FileNotFoundException; class ExceptionExample { public static void main(String[] args) throws FileNotFoundException { FileInputStream dis = new FileInputStream("c:\\" + args[0]); } }
Compliant Solution
To overcome the problem, the exception must be caught while taking special care to sanitize the message before propagating it to the caller. In cases where the exception type itself can reveal too much, consider throwing a different exception (with a different message) altogether.
import java.io.FileInputStream; import java.io.FileNotFoundException; class NewException extends Exception { //common exception for abstraction purposes public NewException() { super("Error!"); } } class ExceptionExample { public static void main(String[] args) throws NewException { try { FileInputStream dis = new FileInputStream("c:\\" + args[0]); } catch(FileNotFoundException fnf) { throw new NewException(); } //sanitized message } }
While following this guideline, make sure that security exceptions such as java.security.AccessControlException
and java.lang.SecurityException
are not swallowed or masked in the process. This can lead to far more pernicious effects.
Risk Assessment
Exceptions may inadvertently reveal sensitive information unless care is taken to limit the information displayed as the result of an exception.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXC01-J |
medium |
probable |
high |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[SCG 07]] Guideline 3-4 Purge sensitive information from exceptions
[[Gong 03]] 9.1 Security Exceptions
[[MITRE 09]] CWE ID 209 "Error Message Information Leak"
EXC00-J. Handle exceptions appropriately 10. Exceptional Behavior (EXC) EXC02-J. Prevent exceptions while logging data