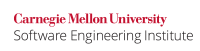
According to the Java API [[API 06]], class java.io.File
:
A pathname, whether abstract or in string form, may be either absolute or relative. An absolute pathname is complete in that no other information is required in order to locate the file that it denotes. A relative pathname, in contrast, must be interpreted in terms of information taken from some other pathname.
An absolute path may sometimes contain aliases, shadows, symbolic links and shortcuts as opposed to canonical paths, which refer to actual files or directories that these point to. These path names must be fully resolved before any file validation operations are performed. For instance, resolving a symbolic link called trace
may yield its actual path on the file system, such as, /home/system/trace
.
The process of canonicalizing file names also makes it easier to verify a path, directory, or file name by making it easier to compare names. This is because extraneous characters are eliminated during canonicalization.
Noncompliant Code Example
This noncompliant code example accepts the file path as a command line argument and uses the getAbsolutePath()
method to obtain the absolute file path. This method does not automatically resolve symbolic links.
Let argv[0]
be the string java/dirname/filename
, where /tmp/java/
is a symbolic link that points to the directory /dirname/
present on the local file system. The application desires to restrict the user from operating on files outside the /tmp
directory and uses a validate()
method to enforce this condition. An adversary who can create symbolic links in /tmp
can cause the program to pass validation checks by supplying the unresolved path. After the validation, any file operations performed are reflected in the file pointed to by the symbolic link. If an attacker enters an unresolved input such as java/dirname/filename
, the validation will pass because the root directory of the compiled path name is still /tmp
, but the operations will be carried out on the file /dirname/filename
.
On Windows and Macintosh systems, this behavior is not observed. The symbolic link, aliases and short cuts are fully resolved on these platforms.
public static void main(String[] args) { File f = new File("/tmp/" + args[0]); String absPath = f.getAbsolutePath(); if(!validate(absPath)) { // Validation throw new IllegalArgumentException(); } }
Compliant Solution
This compliant solution uses the getCanonicalPath()
method, introduced in Java 2, because it resolves the aliases, shortcuts or symbolic links consistently, across all platforms. The value of the alias (if any) is not included in the returned value. Moreover, relative references like the double period (..) are also removed so that the input is reduced to a canonicalized form before validation is carried out. Consequently, this compliant solution is also compliant with IDS18-J. Prevent directory traversal attacks in that, an adversary cannot use ../
sequences to break out of the specified directory when the validate()
method is present.
public static void main(String[] args) throws IOException { File f = new File("/tmp/" + args[0]); String canonicalPath = f.getCanonicalPath(); if(!validate(canonicalPath)) { // Validation throw new IllegalArgumentException(); } }
The getCanonicalPath()
method throws a security exception when used within applets as it reveals too much information about the host machine. The getCanonicalFile()
method behaves like getCanonicalPath()
but returns a new File
object instead of a String
.
Compliant solution
A comprehensive way of handling this issue is to grant the application the permissions to operate on files present only within /tmp
. This can be achieved by specifying the absolute path of the program in the security policy file and granting the java.io.FilePermission
with the target name as /tmp
and the actions as read
and write
. This is shown below.
grant codeBase "file:/home/programpath/" { permission java.io.FilePermission "/tmp", "read, write"; };
The guideline ENV02-J. Create a secure sandbox using a Security Manager contains more information on using a security manager.
Risk Assessment
Using path names from untrusted sources without first canonicalizing the filenames makes it difficult or impossible to validate file names and paths.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO00- J |
medium |
unlikely |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as FIO02-C. Canonicalize path names originating from untrusted sources.
This rule appears in the C++ Secure Coding Standard as FIO02-CPP. Canonicalize path names originating from untrusted sources.
References
[[API 06]] method getCanonicalPath()
[[API 06]] method getCanonicalFile()
[[Harold 99]]
[[MITRE 09]] CWE ID 171 "Cleansing, Canonicalization, and Comparison Errors", CWE ID 647
"Use of Non-Canonical URL Paths for Authorization Decisions"
09. Input Output (FIO) 09. Input Output (FIO) FIO01-J. Do not let Runtime.exec() fail or block indefinitely