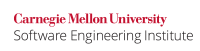
According to Sun's Secure Coding Guidelines [[SCG 2007]]
The (Java) language is type-safe, and the runtime provides automatic memory management and range-checking on arrays. These features also make Java programs immune to the stack-smashing and buffer overflow attacks possible in the C and C++ programming languages, and that has been described as the single most pernicious problem in computer security today.
While this statement is true, arithmetic operations in the Java platform require just as much caution as C and C++ do because integer operations can result in overflow. Java does not provide any indication of overflow conditions and silently wraps. While integer overflows in vulnerable C and C++ programs can result in the execution of arbitrary code; in Java, wrapped values typically result in incorrect computations and unanticipated outcomes.
According to the Java Language Specification, Section 4.2.2, "Integer Operations"
The built-in integer operators do not indicate overflow or underflow in any way. Integer operators can throw a
NullPointerException
if unboxing conversion of anull
reference is required. Other than that, the only integer operators that can throw an exception are the integer divide operator/
and the integer remainder operator%
, which throw anArithmeticException
if the right-hand operand is zero, and the increment and decrement operators ++ and -- which can throw anOutOfMemoryError
if boxing conversion is required and there is not sufficient memory available to perform the conversion.
The integral types in Java are byte
, short
, int
, and long
, whose values are 8-bit, 16-bit, 32-bit and 64-bit signed twoâs-complement integers, respectively, and char
, whose values are 16-bit unsigned integers representing UTF-16 code units.
According to the Java Language Specification, Section 4.2.1, "Integral Types and Values," the values of the integral types are integers in the following ranges:
- For byte, from â“128 to 127, inclusive
- For short, from â“32,768 to 32,767, inclusive
- For int, from â“2,147,483,648 to 2,147,483,647, inclusive
- For long, from â“9,223,372,036,854,775,808 to 9,223,372,036,854,775,807, inclusive
- For char, from
\u0000
to\uffff
inclusive, that is, from 0 to 65,535
The table below shows the integer overflow behavior of the integral operators.
Operator |
Overflow |
|
Operator |
Overflow |
|
Operator |
Overflow |
|
Operator |
Overflow |
---|---|---|---|---|---|---|---|---|---|---|
|
yes |
|
|
yes |
|
|
no |
|
|
no |
|
yes |
|
|
yes |
|
|
no |
|
|
no |
|
yes |
|
|
yes |
|
|
no |
|
|
no |
|
yes |
|
|
no |
|
\ |
no |
|
|
no |
|
no |
|
|
no |
|
|
no |
|
|
no |
|
yes |
|
|
no |
|
|
no |
|
|
no |
|
yes |
|
|
no |
|
|
no |
|
||
|
no |
|
|
no |
|
un |
no |
|
||
|
yes |
|
|
no |
|
un |
yes |
|
Failure to account for integer overflow has resulted in failures of real systems, for example, when implementing the compareTo()
method. The meaning of the return value of the compareTo()
method is defined only in terms of its sign and whether it is zero; the magnitude of the return value is irrelevant. Consequently, an apparent but incorrect optimization would be to subtract the operands and return the result. For operands of opposite signs, this can result in integer overflow, consequently violating the compareTo()
contract [[Bloch 2008, Item 12]].
Overview of Compliant Techniques
The three main techniques for detecting unintended integer overflow are
- Pre-condition testing of the inputs. Check the inputs to each arithmetic operator to ensure that overflow cannot occur. Throw an
ArithmeticException
when the operation would overflow if it were performed; otherwise, perform the operation. We call this technique "Pre-condition the inputs" hereafter, for convenience. - Use a larger type and downcast. Cast the inputs to the next larger primitive integer type and perform the arithmetic in the larger size. Check each intermediate result for overflow of the original smaller type, and throw an
ArithmeticException
if the range check fails. Note that the range check must be performed after each arithmetic operation. Downcast the final result to the original smaller type before assigning to the result variable. This approach cannot be used for typelong
becauselong
is already the largest primitive integer type. - Use
BigInteger
. Convert the inputs into objects of typeBigInteger
and perform all arithmetic usingBigInteger
methods. Throw anArithmeticException
if the final result is outside the range of the original smaller type; otherwise, convert back to the intended result type.
The "Pre-condition the inputs" technique requires different pre-condition tests for each arithmetic operation. This can be somewhat more difficult to understand than either of the other two approaches.
The "Use a larger type and downcast" technique is the preferred approach for the cases to which it applies. The checks it requires are simpler than those of the previous technique; it is substantially more efficient than using BigInteger
.
The "Use BigInteger
" technique is conceptually the simplest of the three techniques. However, it requires the use of method calls for each operation in place of primitive arithmetic operators; this may obscure the intended meaning of the code. This technique will take more time and memory than the other techniques.
Pre-conditioning the Inputs
The following code example shows the necessary pre-conditioning checks required for each arithmetic operation on arguments of type int
. The checks for the other integral types are analogous. In this example, we choose to throw an exception when integer overflow would occur; any other error handling is also acceptable.
static final preAdd(int left, int right) throws ArithmeticException { if (right > 0 ? left > Integer.MAX_VALUE - right : left < Integer.MIN_VALUE - right) { throw new ArithmeticException("Integer overflow"); } } static final preSubtract(int left, int right) throws ArithmeticException { if (right > 0 ? left < Integer.MIN_VALUE + right : left > Integer.MAX_VALUE + right) { throw new ArithmeticException("Integer overflow"); } } static final preMultiply(int left, int right) throws ArithmeticException { if (right>0 ? left > Integer.MAX_VALUE/right || left < Integer.MIN_VALUE/right : (right<-1 ? left > Integer.MIN_VALUE/right || left < Integer.MAX_VALUE/right : right == -1 && left == Integer.MIN_VALUE) ) { throw new ArithmeticException("Integer overflow"); } } static final preDivide(int left, int right) throws ArithmeticException { if ((left == Integer.MIN_VALUE) && (right == -1)) { throw new ArithmeticException("Integer overflow"); } } static final preAbs(int a) throws ArithmeticException { if (a == Integer.MIN_VALUE) { throw new ArithmeticException("Integer overflow"); } } static final preNegate(int a) throws ArithmeticException { if (a == Integer.MIN_VALUE) { throw new ArithmeticException("Integer overflow"); } }
These checks can be simplified when the original type is char
. Because the range of type char
includes only positive values, all comparisons with negative values may be omitted.
Noncompliant Code Example
Either operation in this noncompliant code example could produce a result that overflows the range of int
. When overflow occurs, the result will be incorrect.
public int multAccum(int oldAcc, int newVal, int scale) { // May result in overflow return oldAcc + (newVal * scale); }
Compliant Solution (Pre-Condition the Inputs)
This compliant solution uses the preAdd()
and preMultiply()
methods defined above to indicate errors if the corresponding operations might overflow.
public int multAccum(int oldAcc, int newVal, int scale) throws ArithmeticException { preMultiply(newVal, Scale); final int temp = newVal * scale; preAdd(oldAcc, temp); return oldAcc + temp; }
Compliant Solution (Use a Larger Type and Downcast)
For all integral types other than long
, the next larger integral type can represent the result of any single integral operation. For example, operations on values of type int
can be safely performed using type long
. Therefore, we can perform an operation using the larger type and range-check before downcasting to the original type. Note, however, that this guarantee holds only for a single arithmetic operation; larger expressions without per-operation bounds checking may overflow the larger type.
This approach cannot be applied for type long
because long
is the largest primitive integral type. Use the "Use BigInteger" technique when the original variables are of type long
.
This compliant solution shows the implementation of a method for checking whether a long value falls within the representable range of an int
. The implementations of range checks for the smaller primitive integer types are similar.
public long intRangeCheck(long value) throws ArithmeticOverflow { if ((value < Integer.MIN_VALUE) || (value > Integer.MAX_VALUE)) { throw new ArithmeticException("Integer overflow"); } return value; } public int multAccum(int oldAcc, int newVal, int scale) throws ArithmeticException { final long res = intRangeCheck(((long) oldAcc) + intRangeCheck((long) newVal * (long) scale)); return (int) res; // safe down-cast }
Compliant Solution (Use BigInteger
)
Type BigInteger
is the standard arbitrary-precision integer type provided by the Java standard libraries. The arithmetic operations implemented as methods of this type cannot themselves overflow; instead, they produce the numerically correct result. As a consequence, compliant code performs only a single range checkâ”just before converting the final result to the original smaller type. This property provides conceptual simplicity. An unfortunate consequence of this technique is that compliant code must be written using method calls instead of primitive arithmetic operators; this can obscure the intent of the code. Operations on objects of type BigInteger
can also be significantly less efficient than operations on the original primitive integer type.
private static final BigInteger bigMaxInt = BigInteger.valueOf(Int.MAX_VALUE); private static final BigInteger bigMinInt = BigInteger.valueOf(Int.MIN_VALUE); public BigInteger intRangeCheck(BigInteger val) throws ArithmeticException { if (val.compareTo(bigMaxInt) == 1 || val.compareTo(bigMinInt) == -1) { throw new ArithmeticException("Integer overflow"); } return val; } public int multAccum(int oldAcc, int newVal, int scale) throws ArithmeticException { BigInteger product = BigInteger.valueOf(newVal).multiply(BigInteger.valueOf(scale)); BigInteger res = intRangeCheck(BigInteger.valueOf(oldAcc).add(product)); return res.intValue(); // safe conversion }
Noncompliant Code Example AtomicInteger
Operations on objects of type AtomicInteger
suffer from the same overflow issues as do the other integer types. The solutions are generally similar to those shown above; however, concurrency issues add additional complications. First, potential issues with time-of-check-time-of-use must be avoided. (See guidleine VNA02-J. Ensure that compound operations on shared variables are atomic for more information). Secondly, use of an AtomicInteger
creates happens-before relationships between the various threads that access it. Consequently, changes to the number or order of accesses may alter the execution of the overall program. In such cases you must either choose to accept the altered execution or carefully craft the implementation of your compliant technique to preserve the exact number and order of accesses to the AtomicInteger
.
This noncompliant code example uses an AtomicInteger
, which is part of the concurrency utilities. The concurrency utilities lack integer overflow checks.
class InventoryManager { private final AtomicInteger itemsInInventory = new AtomicInteger(100); //... public final void nextItem() { itemsInInventory++; } }
Consequently, itemsInInventory
may wrap around to Integer.MIN_VALUE
after the increment operation.
Compliant Solution (AtomicInteger
)
This compliant solution uses the get()
and compareAndSet()
methods provided by AtomicInteger
to guarantee successful manipulation of the shared value of itemsInInventory
. Note the following:
- The number and order of accesses to
itemsInInventory
remains unchanged from the noncompliant code example. - All operations on the value of
itemsInInventory
are performed on a temporary local copy of its value. - The overflow check in this example is performed in inline code, rather than encapsulated in a method call. This is an acceptable alternative implementation. The choice of method call vs. inline code should be made according to your organization's standards and needs.
class InventoryManager { private final AtomicInteger itemsInInventory = new AtomicInteger(100); public final void nextItem() { while (true) { int old = itemsInInventory.get(); if (old == Integer.MAX_VALUE) { throw new ArithmeticException("Integer overflow"); } int next = old + 1; // Increment if (itemsInInventory.compareAndSet(old, next)) { break; } } // end while } // end removeItem() }
The arguments to the compareAndSet()
method are the expected value of the variable when the method is invoked and the intended new value. The variable's value is updated if, and only if, the current value and the expected value are equal (see [[API 2006]] class AtomicInteger
). Refer to guideline VNA02-J. Ensure that compound operations on shared variables are atomic for more details.
Exceptions
INT00-EX1: Depending on circumstances, integer overflow could be benign. For instance, the Object.hashcode()
method could return all representable values of type int
. Furthermore, many algorithms for computing hashcodes intentionally allow overflow to occur.
INT00-EX2: The added complexity and cost of programmer-written overflow checks may exceed their value for all but the most critical code. In such cases, consider the alternative of treating integral values as though they are tainted data, using appropriate range checks as the sanitizing code. These range checks should ensure that incoming values cannot cause integer overflow. Note that sound determination of allowable ranges may require deep understanding of the details of the code protected by the range checks; correct determination of the allowable ranges may be extremely difficult.
Risk Assessment
Failure to perform appropriate range checking can lead to integer overflows, which can cause unexpected program control flow or unanticipated program behavior.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT00-J |
medium |
unlikely |
medium |
P4 |
L3 |
Automated Detection
Automated detection of integer operations that can potentially overflow is straightforward. Automatic determination of which potential overflows are true errors and which are intended by the programmer is infeasible. Heuristic warnings could be helpful.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
C Secure Coding Standard: INT32-C. Ensure that operations on signed integers do not result in overflow
C++ Secure Coding Standard: INT32-CPP. Ensure that operations on signed integers do not result in overflow
MITRE CWE: CWE-682 "Incorrect Calculation"
MITRE CWE: CWE-190 "Integer Overflow or Wraparound"
MITRE CWE: CWE-191 "Integer Underflow (Wrap or Wraparound)"
Bibliography
[[API 2006]] class AtomicInteger
[[Bloch 2005]] Puzzle 27: Shifty i's[[SCG 2007]] Introduction
[[JLS 2005]] Section 4.2.2 "Integer Operations," Section 15.22
"Bitwise and Logical Operators"
[[Seacord 2005]] Chapter 5. Integers
[[Tutorials 2008]] Primitive Data Types
06. Integers (INT) 06. Integers (INT) INT01-J. Check ranges before casting integers to narrower types