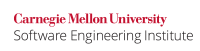
John von Neumann's quote is widely known:
Anyone who considers arithmetical methods of producing random digits is, of course, in a state of sin.
Pseudorandom number generators (PRNGs) use deterministic mathematical algorithms to produce a sequence of numbers with good statistical properties, but the numbers produced are not genuinely random. PRNGs usually start with an arithmetic seed value. The algorithm uses this seed to generate an output value and a new seed as well, which is used to generate the next value, and so on.
The Java API provides a PRNG, the java.util.Random
class. This PRNG is portable and repeatable. Consequently, if two random instances are created using the same seed, they generate identical sequences of numbers in all Java implementations. Sometimes the same seed is reused on application initialization or after every system reboot. At other times, the current time obtained from the system clock is used to derive the seed. An adversary can learn the value of the seed by performing some reconnaissance on the remote server and proceed to build a lookup table for estimating future seed values.
Noncompliant Code Example
If the same seed value is used, the same sequence of numbers is obtained; as a result, the numbers are not "random".
import java.util.Random; // ... Random number = new Random(123L); //... for (int i=0; i<20; i++) { // generate another random integer in the range [0, 20] int n = number.nextInt(21); System.out.println(n); }
There are cases where the same sequence of random numbers is desirable, such as when running regression tests of program behavior. In other applications, generating the same sequence of random numbers may expose a vulnerability.
Compliant Solution
This compliant solution uses the java.security.SecureRandom
class to produce high quality random numbers.
import java.security.SecureRandom; import java.security.NoSuchAlgorithmException; // ... public static void main (String args[]) { try { SecureRandom number = SecureRandom.getInstance("SHA1PRNG"); // Generate 20 integers 0..20 for (int i = 0; i < 20; i++) { System.out.println(number.nextInt(21)); } } catch (NoSuchAlgorithmException nsae) { // Forward to handler } }
Exceptions
MSC30-EX1: Using a null
seed value (as opposed to reusing it) may improve security marginally but should only be used for non-critical applications. Java's default seed uses the system's time in milliseconds. This exception is not recommended for applications requiring high security (for instance, session IDs should be adequately random). When used, explicit documentation of this exception is encouraged.
import java.util.Random; // ... Random number = new Random(); int n; //... for (int i=0; i<20; i++) { // Re-seed generator number = new Random(); // Generate another random integer in the range [0, 20] n = number.nextInt(21); System.out.println(n); }
For noncritical cases, such as adding some randomness to a game, the Random
class is considered fine. However, it is worth reiterating that the resulting low entropy random numbers are not random enough to be used for more serious applications, such as cryptography.
Risk Assessment
Predictable random number sequences can weaken the security of critical applications such as cryptography.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC30- J |
high |
probable |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as MSC30-C. Do not use the rand() function for generating pseudorandom numbers.
This rule appears in the C++ Secure Coding Standard as MSC30-CPP. Do not use the rand() function for generating pseudorandom numbers.
References
[API 06] Class Random
[API 06] Class SecureRandom
[Find Bugs 08] BC: Random objects created and used only once
[[Monsch 06]]
[[MITRE 09]] CWE ID 330 "Use of Insufficiently Random Values", CWE ID 327
, "Use of a Broken or Risky Cryptographic Algorithm," CWE ID 330
, "Use of Insufficiently Random Values", CWE ID 333
"Failure to Handle Insufficient Entropy in TRNG", CWE ID 332
"Insufficient Entropy in PRNG", CWE ID 337
"Predictable Seed in PRNG", CWE ID 336
"Same Seed in PRNG"
MSC09-J. Do not use insecure or weak cryptographic algorithms 49. Miscellaneous (MSC) MSC31-J. Never hardcode sensitive information