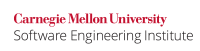
When an API (see table below) is invoked on a Class
object, a comparison is run between the immediate caller's class loader and that of the Class
object. The Class
object is the object on which an API is invoked. According to [[JLS 05]]:
The method getClass returns the Class object that represents the class of the object.
APIs capable of bypassing SecurityManager's checks |
---|
|
|
|
|
|
|
|
|
|
|
|
|
|
Security manager checks may get bypassed depending on the immediate caller's class loader.
For instance, in the presence of a security manager, the getSystemClassLoader
and getParent
methods succeed only if the caller's class loader is the delegation ancestor of the current class loader or if the caller's class loader is the same as the current one or if the code in the current execution context has the RunTimePermission
, namely "getClassLoader
".
Noncompliant Code Example
The createInstance
method is the immediate caller of java.lang.Class.newInstance
in this noncompliant example. The newInstance
method is being invoked on the dateClass
Class
object. The issue is that the untrustedCode
method can trigger the instantiation of a new class even though it should not have the permission to do so. This behavior is not caught by the security manager.
public class ExceptionExample { public static void untrustedCode() { Date now = new Date(); Class<?> dateClass = now.getClass(); createInstance(dateClass); } public static void createInstance(Class<?> dateClass) { try { // Create another Date object using the Date Class Object o = dateClass.newInstance(); if (o instanceof Date) { Date d = (Date)o; System.out.println("The time is: " + d.toString()); } } catch (InstantiationException ie) { System.out.println(ie.toString()); } catch (IllegalAccessException iae) { System.out.println(iae.toString()); } } }
A related issue is described in SEC03-J. Do not allow tainted parameters while using APIs that perform access checks against the immediate caller.
Compliant Solution
Do not accept Class
, ClassLoader
or Thread
instances from untrusted code. If inevitable, safely acquire these instances by ensuring they come from trusted sources. Additionally, make sure to discard tainted inputs from untrusted code. Likewise, objects returned by the affected methods should not be propagated back to the untrusted code.
Note that the Class.newInstance()
} method requires the class to contain a no-argument constructor. If this requirement is not satisfied, a runtime exception results, which indirectly prevents a security breach.
Risk Assessment
Bypassing Securitymanager
checks may seriously compromise the security of a Java application.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SEC33-J |
high |
probable |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Gong 03]] Section 4.3.2, Class Loader Delegation Hierarchy
[[SCG 07]] Guideline 6-2 Safely invoke standard APIs that bypass SecurityManager checks depending on the immediate caller's class loader
SEC31-J. Guard doPrivileged blocks against untrusted invocations 01. Platform Security (SEC) SEC03-J. Do not expose standard APIs that use the immediate caller's class loader instance to untrusted code