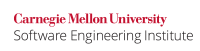
A Serializable
class can overload the
method, which is called when an object of that class is being deserialized. Both this method and the method Serializable
.readObject()readResolve()
must treat the serialized data as potentially malicious and must refrain from performing potentially dangerous operations, unless the programmer has expressly indicated for the particular deserialization at hand that the class should be permitted to perform potentially dangerous operations.
This rule complements rule SER12-J. Prevent deserialization of untrusted classes. Whereas SER12-J requires the programmer to ensure the absence of classes that might perform dangerous operations by validating data before deserializing it, SER13-J requires that all serializable classes refrain, by default, from performing dangerous operations during deserialization. SER12-J and SER13-J both guard against the same class of deserialization vulnerabilities. Theoretically, a given system is secure against this class of vulnerabilities if either (1) all deployed code on that system follows SER12-J or (2) all deployed code on that system follows SER13-J. However, because much existing code violates these rules, the CERT Coding Standard takes the "belt and suspenders" approach and requires compliant code to follow both rules.
Non-Compliant Code Example
The class OpenedFile
in the following non-compliant code example opens a file during deserialization. Operating systems typically impose a limit on the number of open file handles per process; this limit typically is not large (e.g., 1024). Consequently, deserializing a list of OpenedFile
objects can consume all file handles available to the process and consequently cause the program to malfunction if it attempts to open another file before the deserialized OpenedFile
objects get garbage-collected.
import java.io.*; class OpenedFile implements Serializable { public String filename; public BufferedReader reader; public OpenedFile(String _filename) throws FileNotFoundException { filename = _filename; init(); } private void init() throws FileNotFoundException { reader = new BufferedReader(new FileReader(filename)); } private void writeObject(ObjectOutputStream out) throws IOException { out.writeUTF(filename); } private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { filename = in.readUTF(); init(); } }
Compliant Solution
In this compliant solution, the readObject()
method throws an exception unless the potentially dangerous class was whitelisted in the manner used for the compliant solution of SER12-J. Prevent deserialization of untrusted classes. The reasoning is that a programmer who expressly whitelists a class for deserialization can reasonably be presumed to be aware of what the whitelisted class does during deserialization. For example, for a web app, whitelisting the above OpenedFile
class might be considered an acceptable risk when deserializing data from an authenticated adminstrator but an unacceptable risk when deserializing data from an untrusted Internet user.
Note that the below compliant solution for SER13-J is different from and complementary to the compliant solution in SER12-J. In the compliant solution for SER12-J, the source code location that invokes deserialization is modified to use a custom subclass of ObjectInputStream
. This subclass overrides the resolveClass
method to check whether class of the serialized object is whitelisted before that class's readObject
method gets called. In contrast, in the compliant solution below for SER13-J, the whitelist is checked inside the readObject
method of the dangerous serializable class. When both solutions are used together, a minor downside is that there is some redundancy, since the whitelist is checked twice (once before readObject
, and once during readObject
) even though a single check would have sufficed. However, the upside is that it now requires mistakes in two different places of the code to yield an exploitable vulnerability.
import java.io.*; import java.lang.reflect.*; class OpenedFile implements Serializable { public String filename; public BufferedReader reader; public OpenedFile(String _filename) throws FileNotFoundException { filename = _filename; init(); } private void init() throws FileNotFoundException { reader = new BufferedReader(new FileReader(filename)); } private void writeObject(ObjectOutputStream out) throws IOException { out.writeUTF(filename); } private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { boolean isWhitelisted = false; try { Object whitelist = in.getClass().getDeclaredField("whitelist").get(in); Method contains = whitelist.getClass().getMethod("contains", new Class[]{Object.class}); isWhitelisted = contains.invoke(whitelist, this.getClass().getName()).equals(Boolean.TRUE); } catch (ReflectiveOperationException e) {} if (!isWhitelisted) { throw new SecurityException("Attempted to deserialize unexpected class."); } filename = in.readUTF(); init(); } }
Risk Assessment
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
SER13-J | High | Likely | High | P9 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Useful for developing exploits that detect violation of this rule |
Related Guidelines
Bibliography