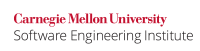
Java's file-manipulation functions often indicate failure with a return value, rather than throwing an exception. The Java Tutorial for Java 7 notes:
Prior to the Java SE 7 release, the
java.io.File
class was the mechanism used for file I/O, but it had several drawbacks.Many methods didn't throw exceptions when they failed, so it was impossible to obtain a useful error message. For example, if a file deletion failed, the program would receive a "delete fail" but wouldn't know if it was because the file didn't exist, the user didn't have permissions, or there was some other problem.
Consequently, it is easy for file operations to fail silently, if the methods' return values are ignored. Therefore, do not ignore return values of file-based methods. (This rule is a specific instance of EXP00-J. Do not ignore values returned by methods.)
Noncompliant Code Example (delete()
)
This noncompliant code example attempts to delete the file specified, but gives no indication of its success. The [API 2006] defines File.delete()
to only throw a SecurityException
, if the program is not authorized to delete the file. No other exceptions are thrown; so it is easy for the deletion to fail, with no indication of why.
File file = /* initialize */ file.delete();
Compliant Solution
This compliant solution checks the return value of delete()
.
File file = new File(args[0]); if (!file.delete()) { System.out.println("Deletion failed"); }
Compliant Solution (Java 1.7)
This compliant solution uses the Files.delete()
method from Java 1.7 to delete the file. [[J2SE 2011]] defines Files.delete()
to throw the following exceptions:
Exception |
Reason |
---|---|
|
if the file does not exist |
|
if the file is a directory and could not otherwise be deleted because the directory is not empty |
|
if an I/O error occurs |
|
In the case of the default provider, and a security manager is installed, the SecurityManager.checkDelete(String) method is invoked to check delete access to the file |
Path file = new File("file").toPath(); try { Files.delete(file); } catch (IOException x) { // handle error }
Risk Assessment
Failure to check file operation errors can result in unexpected behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO09-J |
medium |
probable |
high |
P4 |
L3 |
Automated Detection
TODO
Related Guidelines
Bibliography
[[API 2006]] File.delete()
[[J2SE 2011]] Files.delete()
[[Seacord 2005a]] Chapter 7, "File I/O"
FIO08-J. Do not log sensitive information outside a trust boundary 12. Input Output (FIO) FIO10-J. Do not let external processes block on IO buffers