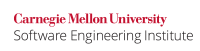
Creating a file with insufficiently restrictive access permissions may allow an unprivileged user to access that file. Although access permissions are heavily dependent on the file system, many file-creation functions provide mechanisms to set (or at least influence) access permissions. When these functions are used to create files, appropriate access permissions should be specified to prevent unintended access. Also, when setting access permissions, it is important to make sure that an attacker is not able to alter them.
Noncompliant Code Example
The constructors for FileOutputStream
and FileWriter
do not allow the programmer to explicitly specify file access permissions. In this noncompliant code example, if the constructor creates a new file, the access permissions are implementation-defined.
Writer out = new FileWriter("file");
Implementation Details (POSIX)
On POSIX-compliant systems, the permissions may be restricted by the value of the POSIX C umask()
function [[Open Group 2004]].
The operating system modifies the access permissions by computing the intersection of the inverse of the umask and the permissions requested by the process [[Viega 2003]]. For example, if the variable requested_permissions
contained the permissions passed to the operating system to create a new file, the variable actual_permissions
would be the actual permissions that the operating system would use to create the file:
requested_permissions = 0666; actual_permissions = requested_permissions & ~umask();
For OpenBSD and Linux operating systems, any file created will have mode S_IRUSR|S_IWUSR|S_IRGRP|S_IWGRP|S_IROTH|S_IWOTH
(0666), as modified by the process's umask value. (See fopen(3)
in the OpenBSD Manual Pages [[OpenBSD]].)
Compliant Solution (POSIX, Java 1.7)
The Files.newByteChannel()
method allows a file to be created with specific permissions. This method is platform-independent, although the permissions are platform-specific.
Path file = new File("file").toPath(); // Throw exception rather than overwrite existing file Set<OpenOption> options = new HashSet<OpenOption>(); options.add(StandardOpenOption.CREATE_NEW); options.add(StandardOpenOption.APPEND); // File permissions should be that only user may read/write file Set<PosixFilePermission> perms = PosixFilePermissions.fromString("rw-------"); FileAttribute<Set<PosixFilePermission>> attr = PosixFilePermissions.asFileAttribute(perms); try (SeekableByteChannel sbc = Files.newByteChannel(file, options, attr)) { // write data };
Exceptions
FIO03-EX0: This guideline is not required for files created in secure directories, although it is still a good idea.
FIO03-EX1: This guideline is not required for files that do not contain sensitive information.
Risk Assessment
The ability to determine if an existing file has been opened or a new file has been created provides greater assurance that a file other than the intended file is not acted upon.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO03-J |
medium |
probable |
high |
P4 |
L3 |
Automated Detection
TODO
Related Guidelines
CERT C++ Secure Coding Standard: FIO06-CPP. Create files with appropriate access permissions
CERT C Secure Coding Standard: FIO06-C. Create files with appropriate access permissions
MITRE CWE: CWE-279, "Insecure Execution-assigned Permissions"
MITRE CWE: CWE-276, "Insecure Default Permissions"
MITRE CWE: CWE-732, "Incorrect Permission Assignment for Critical Resource"
Bibliography
[[API 2006]]
[[CVE]]
[[J2SE 2011]]
[[OpenBSD]]
[[Open Group 2004]] "The open
function," and "The umask
function"
[[Viega 2003]] Section 2.7, "Restricting Access Permissions for New Files on UNIX"
[[Dowd 2006]] Chapter 9, "UNIX 1: Privileges and Files"
FIO01-J. Do not expose buffers created using the wrap() or duplicate() methods to untrusted code 12. Input Output (FIO) FIO05-J. Do not create multiple buffered wrappers on a single InputStream