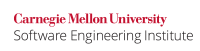
If data members are declared public or protected, it is difficult to control how they are accessed. It is possible that they could be manipulated in unintented ways, with undefined consequences. Better is to declare all data members private and define accessor functions that control their uses to those intended. Also, modification of data members can be monitored as appropriate (e.g., by logging). Public modifier functions should preserve any invariants of the type.
Noncompliant Code Example
public class Widget { public int total; // ... void add (someType someParameters) { // ... total++; // ... } void remove (someType someParameters) { // ... total--; // ... } // ... }
In this example, the data member total
is meant to keep track of the total number of elements as they are added and removed. However, as a public data member, it can be altered by any other part of the system independently of those actions.
Compliant Solution
public class Widget { private int total; // ... void add (someType someParameters) { // ... total++; // ... } void remove (someType someParameters) { // ... total--; // ... } // ... public int getTotal () { return total; } // ... }
Now, total
is private, and the functions that modify its value preserve any class invariants.
Risk Assessment
Not properly managing resources could lead to an attacker causing unintended behavior.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ00-J |
medium |
likely |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as OBJ00-CPP. Declare data members private.
References
[[JLS 06]] Section 6.6, Access Control
[[SCG 07]] Guideline 3-2 Define wrapper methods around modifiable internal state
[[Long 05]] Section 2.2, Public Fields
[[Bloch 08]] Items 13: Minimize the accessibility of classes and members; 14: In public classes, use accessor methods, not public fields
07. Object Orientation (OBJ) 07. Object Orientation (OBJ) OBJ01-J. Understand how a superclass can affect a subclass