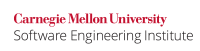
It is important to disallow operations on tainted inputs in a doPrivileged()
block. This is because an adversary may supply malicious input that may result in privilege escalation attacks.
Noncompliant Code Example
This noncompliant code example accepts a tained filename
argument. An adversary may supply the name of a sensitive password file, complete with the path and consequently force operations to be performed on the wrong file.
private void privilegedMethod(final String filename) throws FileNotFoundException { try { FileInputStream fis = (FileInputStream) AccessController.doPrivileged( new PrivilegedExceptionAction() { public FileInputStream run() throws FileNotFoundException { return new FileInputStream(filename); } } ); // do something with the file and then close it } catch (PrivilegedActionException e) { // forward to handler and log } }
Compliant Solution
This compliant solution explicitly hardcodes the name of the file and confines the variables used in the privileged block to the same method. This ensures that no malicious file can be loaded by exploiting the privileges of the corresponding code.
private void privilegedMethod() throws FileNotFoundException { try { FileInputStream fis = (FileInputStream) AccessController.doPrivileged( new PrivilegedExceptionAction() { public FileInputStream run() throws FileNotFoundException { return new FileInputStream("/usr/home/filename"); } } ); // do something with the file and then close it } catch (PrivilegedActionException e) { // forward to handler and log } }
Risk Assessment
Allowing tainted inputs in privileged operations can lead to privilege escalation attacks.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SEC03-J |
high |
likely |
low |
P27 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[API 2006]] method doPrivileged()
[[Gong 2003]] Sections 6.4, AccessController and 9.5 Privileged Code
[[SCG 2007]] Guideline 6-1 Safely invoke java.security.AccessController.doPrivileged
[[MITRE 2009]] CWE ID 266 "Incorrect Privilege Assignment", CWE ID 272
"Least Privilege Violation"
SEC02-J. Guard doPrivileged blocks against untrusted invocations 02. Platform Security (SEC) SEC04-J. Do not expose standard APIs that may bypass Security Manager checks to untrusted code