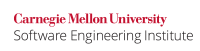
Composite operations on shared variables (consisting of more than one discrete operation) must be performed atomically. Errors can arise from composite operations that need to be perceived atomically but are not [[JLS 05]]. For example, any assignment that depends on an existing value is a composite operation, for example, a = b
. Increment +
and decrement +
operators depend modify the value of the operand based on the existing value of the operand and are consequently always composite operations.
For atomicity of a grouping of calls to independently atomic methods of the existing Java API, see CON07-J. Do not assume that a grouping of calls to independently atomic methods is atomic.
The Java Language Specification also permits reads and writes of 64-bit values to be non-atomic though this is not an issue with most modern JVMs (see CON25-J. Ensure atomicity when reading and writing 64-bit values).
Noncompliant Code Example (increment/decrement)
Pre- and post-increment and pre- and post-decrement operations in Java are non-atomic in that the value written depends upon the value initially read from the operand. For example, x++
is non-atomic because it is a composite operation consisting of three discrete operations: reading the current value of x
, adding one to it, and writing the new, incremented value back to x
.
This noncompliant code example contains a data race that may result in the itemsInInventory
field failing to account for removed items.
class InventoryManager { private static final int MIN_INVENTORY = 3; private int itemsInInventory = 100; public final void removeItem() { if (itemsInInventory <= MIN_INVENTORY) { throw new IllegalStateException("Under stocked"); } itemsInInventory--; } }
For example, if the removeItem()
method is concurrently invoked by two threads, t1 and t2, the execution of these threads may be interleaved so that:
Time |
itemsInInventory= |
Thread |
Action |
---|---|---|---|
1 |
100 |
t1 |
reads the current value of |
2 |
100 |
t2 |
reads the current value of |
3 |
100 |
t1 |
decrements the temporary variable to 99 |
4 |
100 |
t2 |
decrements the temporary variable to 99 |
5 |
99 |
t1 |
writes the temporary variable value to |
6 |
99 |
t2 |
writes the temporary variable value to |
As a result, the effect of the call by t1 is not reflected in itemsInInventory
; the program behaves as if the call was never made.
Noncompliant Code Example (volatile)
This noncompliant code example attempts to resolve the problem by declaring itemsInInventory
volatile.
class InventoryManager { private static final int MIN_INVENTORY = 3; private volatile int itemsInInventory = 100; public final void removeItem() { if (itemsInInventory <= MIN_INVENTORY) { throw new IllegalStateException("under stocked"); } itemsInInventory--; } }
Volatile variables are unsuitable when more than one read/write operation needs to be atomic. The use of a volatile variable in this noncompliant code example guarantees that once itemsInInventory
has been updated, the new value is visible to all threads that read the field. However, because the post decrement operator is nonatomic, even when volatile
is used, the interleaving described in the previous noncompliant code example is still possible.
Compliant Solution (java.util.concurrent.atomic
classes)
The java.util.concurrent
utilities can be used to atomically manipulate a shared variable. This compliant solution defines intemsInInventory
as a java.util.concurrent.atomic.AtomicInteger
variable, allowing composite operations to be performed atomically.
class InventoryManager { private static final int MIN_INVENTORY = 3; private final AtomicInteger itemsInInventory = new AtomicInteger(100); public final void removeItem() { for (;;) { int old = itemsInInventory.get(); if (old > MIN_INVENTORY) { int next = old - 1; // Decrement if(itemsInInventory.compareAndSet(old, next)) { break; } } else { throw new IllegalStateException("Under stocked"); } } // end for } // end removeItem() }
Note that updates to shared atomic variables are visible to other threads.
The compareAndSet()
method takes two arguments, the expected value of a variable when the method is invoked and the updated value. This compliant solution uses this method to atomically set the value of itemsInInventory
to the updated value if and only if the current value equals the expected value [[API 06]]. The for
loop ensures that the removeItem()
method succeeds in decrementing the most recent value of itemsInInventory
as long as the inventory count is greater than MIN_INVENTORY
.
Compliant Solution (method synchronization)
Synchronization provides a way to safely share object state across multiple threads without the need to reason about reorderings, compiler optimizations, and hardware specific behavior.
This compliant solution uses method synchronization to synchronize access to itemsInInventory
. Consequently, access to itemsInInventory
is mutually exclusive and its state consistent across all threads.
class InventoryManager { private static final int MIN_INVENTORY = 3; private int itemsInInventory = 100; public final synchronized void removeItem() { if (itemsInInventory <= MIN_INVENTORY) { throw new IllegalStateException("Under stocked"); } itemsInInventory--; } }
If code is synchronized correctly, updates to shared variables are instantly made visible to other threads. Synchronization is more expensive than using the optimized java.util.concurrent
utilities and should generally be preferred when it is sufficiently complex to carry out the operation atomically using the utilities. When using synchronization, care must be taken to avoid deadlocks (see CON12-J. Avoid deadlock by requesting and releasing locks in the same order).
Compliant Solution (block synchronization)
Constructors and methods can use block synchronization as an alternative to method synchronization. Block synchronization synchronizes a block of code rather than a method, as shown in this compliant solution.
class InventoryManager { private static final int MIN_INVENTORY = 3; private int itemsInInventory = 100; private final Object lock = new Object(); public final synchronized void removeItem() { synchronized(lock) { if (itemsInInventory <= MIN_INVENTORY) { throw new IllegalStateException("Under stocked"); } itemsInInventory--; } } }
Block synchronization is preferable over method synchronization because it reduces the duration for which the lock is held and also protects against denial of service attacks. Block synchronization does not require synchronizing on an internal private lock object instead of the intrinsic lock of the class's object. However, it is more secure to synchronize on an internal private lock object instead of a more accessible lock object (see CON04-J. Use the private lock object idiom instead of the Class object's intrinsic locking mechanism).
Compliant Solution (ReentrantLock
)
This compliant solution uses a java.util.concurrent.locks.ReentrantLock
to atomically perform the post-decrement operation.
class InventoryManager { private static final int MIN_INVENTORY = 3; private int itemsInInventory = 100; private final Lock lock = new ReentrantLock(); public final synchronized void removeItem() { if(lock.tryLock()) { try { if (itemsInInventory <= MIN_INVENTORY) { throw new IllegalStateException("Under stocked"); } itemsInInventory--; } finally { lock.unlock(); } } } // end removeItem() }
Code that uses this lock behaves similar to synchronized code that uses the traditional monitor lock. ReentrantLock
provides several other capabilities, for instance, the tryLock()
method does not block waiting if another thread is already holding the lock. The class java.util.concurrent.locks.ReentrantReadWriteLock
can be used when some thread requires a lock to write information while other threads require the lock to concurrently read the information.
Noncompliant Code Example (addition, volatile fields)
In this noncompliant code example, the two fields a
and b
may be set by multiple threads, using the setValues()
method.
private volatile int a; private volatile int b; public int getSum() throws ArithmeticException { // Check for integer overflow if( b > 0 ? a > Integer.MAX_VALUE - b : a < Integer.MIN_VALUE - b ) { throw new ArithmeticException("Not in range"); } return a + b; } public void setValues(int a, int b) { this.a = a; this.b = b; }
The getSum()
method may return a different sum every time it is invoked from different threads. For instance, if a
and b
currently have the value 0, and one thread calls getSum()
while another calls setValues(1, 1)
, then getSum()
might return 0, 1, or 2. Of these, the value 1 is unacceptable; it is returned when the first thread reads a
and b
, after the second thread has set the value of a
but before it has set the value of b
.
Noncompliant Code Example (addition, atomic integer fields)
The issues described in the previous noncompliant code example can also arise when the volatile variables a
and b
are replaced with atomic integers.
private final AtomicInteger a = new AtomicInteger(); private final AtomicInteger b = new AtomicInteger(); public int getSum() throws ArithmeticException { // Check for integer overflow if( b.get() > 0 ? a.get() > Integer.MAX_VALUE - b.get() : a.get() < Integer.MIN_VALUE - b.get() ) { throw new ArithmeticException("Not in range"); } return a.get() + b.get(); // or, return a.getAndAdd(b.get()); } public void setValues(int a, int b) { this.a.set(a); this.b.set(b); }
For example, when a thread is executing setValues()
another may invoke getSum()
and retrieve an incorrect result. Furthermore, in the absence of synchronization, there are data races in the check for integer overflow.
Compliant Solution (addition)
This compliant solution synchronizes the setValues()
method so that the entire operation is atomic.
private int a; private int b; public synchronized int getSum() throws ArithmeticException { // Check for integer overflow if( b > 0 ? a > Integer.MAX_VALUE - b : a < Integer.MIN_VALUE - b ) { throw new ArithmeticException("Not in range"); } return a + b; } public synchronized void setValues(int a, int b) { this.a = a; this.b = b; }
Unlike the noncompliant code example, if a
and b
currently have the value 0, and one thread calls getSum()
while another calls setValues(1, 1)
, getSum()
may return return 0, or 2, depending on which thread obtains the intrinsic lock first. The locking guarantees that getSum()
will never return the unacceptable value 1.
Risk Assessment
If operations on shared variables are not atomic, unexpected results may be produced. For example, there can be inadvertent information disclosure as one user may be able to receive information about other users.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON01- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class AtomicInteger
[[JLS 05]] Chapter 17, Threads and Locks, section 17.4.5 Happens-before Order, section 17.4.3 Programs and Program Order, section 17.4.8 Executions and Causality Requirements
[[Tutorials 08]] Java Concurrency Tutorial
[[Lea 00]] Sections, 2.2.7 The Java Memory Model, 2.2.5 Deadlock, 2.1.1.1 Objects and locks
[[Bloch 08]] Item 66: Synchronize access to shared mutable data
[[Daconta 03]] Item 31: Instance Variables in Servlets
[[JavaThreads 04]] Section 5.2 Atomic Variables
[[Goetz 06]] 2.3. "Locking"
[[MITRE 09]] CWE ID 667 "Insufficient Locking", CWE ID 413
"Insufficient Resource Locking", CWE ID 366
"Race Condition within a Thread", CWE ID 567
"Unsynchronized Access to Shared Data"
11. Concurrency (CON) 11. Concurrency (CON) CON02-J. Always synchronize on the appropriate object