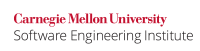
The conditional operator ?:
uses the boolean
value of one expression to decide which of the other two expressions should be evaluated (see JLS, Section 15.25, "Conditional Operator ? :
".)
The general form of a Java conditional expression is operand1 ? operand2 : operand3
.
- If the value of the first operand (
operand1
) istrue
, then the second operand expression (operand2
) is chosen. - If the value of the first operand is
false
, then the third operand expression (operand3
) is chosen.
The conditional operator is syntactically right-associative; for example, a?b:c?d:e?f:g
is equivalent to a?b:(c?d:(e?f:g))
.
The JLS-defined rules for determining the type of the result of a conditional expression (tabulated below) are complicated; programmers could be surprised by the type conversions required for expressions they have written.
Result type determination begins from the top of the table; the compiler applies the first matching rule. The "Operand 2" and "Operand 3" columns refer to operand2
and operand3
(from the above definition), respectively. In the table, constant int
refers to constant expressions of type int
(such as '0' or variables declared final
).
Operand 2 |
Operand 3 |
Resultant type |
---|---|---|
type T |
type T |
type T |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
other numeric |
other numeric |
promoted type of the 2nd and 3rd operands |
T1 = boxing conversion (S1) |
T2 = boxing conversion(S2) |
apply capture conversion to lub(T1,T2) |
See JLS, Section 5.1.7, "Boxing Conversion"; JLS, Section 5.1.10, "Capture Conversion"; and JLS, Section 15.12.2.7, "Inferring Type Arguments Based on Actual Arguments" for additional information on the final table entry.
The complexity of the rules that determine the result type of a conditional expression can lead to unintended type conversions. Consequently, the second and third operands of each conditional expression should always have the same type. This recommendation also applies to boxed primitives.
Noncompliant Code Example
This noncompliant code example prints the value of alpha
as A
, which is of the char
type. The third operand is a constant expression of type int
, whose value 0
can be represented as a char
; numeric promotion is unnecessary. However, this behavior depends on the particular value of the constant integer expression; changing that value can lead to different behavior.
public class Expr { public static void main(String[] args) { char alpha = 'A'; System.out.print(true ? alpha : 0); } }
Compliant Solution
This compliant solution uses identical types for the second and third operands of the conditional expression; the explicit cast clarifies the expected type.
public class Expr { public static void main(String[] args) { char alpha = 'A'; // Cast 0 as a char to explicitly state that the type of the // conditional expression should be char. System.out.print(true ? alpha : ((char) 0)); } }
Noncompliant Code Example
This noncompliant example prints {{65}}â”the ASCII equivalent of A
, instead of the expected A
, because the second operand (alpha
) must be promoted to type int
. The numeric promotion occurs because the value of the third operand (the constant expression '123456') is too large to be represented as a char
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; System.out.print(true ? alpha : 123456); } }
Compliant Solution
The compliant solution explicitly states the intended result type by casting alpha
to type int
. Casting 123456
to type char
would ensure that both operands of the conditional expression have the same type, resulting in A
being printed. However, it would result in data loss when an integer larger than Character.MAX_VALUE
is downcast to type char
. This compliant solution avoids potential truncation by casting alpha
to type int
, the wider of the operand types.
public class Expr { public static void main(String[] args) { char alpha = 'A'; // Cast alpha as an int to explicitly state that the type of the // conditional expression should be int. System.out.print(true ? ((int) alpha) : 123456); } }
Noncompliant Code Example
This noncompliant code example prints 65
instead of A
. The third operand is a variable of type int
, so the second operand (alpha
) must be converted to type int
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0; System.out.print(true ? alpha : i); } }
Compliant Solution
This compliant solution declares i
as type char
, ensuring that the second and third operands of the conditional expression have the same type.
public class Expr { public static void main(String[] args) { char alpha = 'A'; char i = 0; //declare as char System.out.print(true ? alpha : i); } }
Noncompliant Code Example
This noncompliant code example uses boxed and unboxed primitives of different types in the conditional expression. Consequently, the Integer
object is auto-unboxed to its primitive type int
and then converted to the primitive type float
, resulting in loss of precision [[Findbugs 2008]].
public class Expr { public static void main(String[] args) { Integer i = Integer.MAX_VALUE; float f = 0; System.out.print(true ? i : f); } }
Compliant Solution
This compliant solution declares both the operands as Integer
.
public class Expr { public static void main(String[] args) { Integer i = Integer.MAX_VALUE; Integer f = 0; //declare as Integer System.out.print(true ? i : f); } }
Risk Assessment
When the second and third operands of a conditional expression have different types, they can be subject to type conversions that were not anticipated by the programmer.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP14-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
Automated detection of condition expressions whose second and third operands are of different types is straightforward.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[Bloch 2005]] Puzzle 8: Dos Equis
[[Findbugs 2008]] "Bx: Primitive value is unboxed and coerced for ternary operator"
[[JLS 2005]] Section 15.25, "Conditional Operator
? :
"