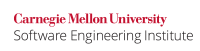
Programmers frequently make errors regarding the precedence rules of operators due to the unintuitive low-precedence levels of &
, |
, ^
, <<
, and >>
. Mistakes regarding precedence rules can be avoided by the suitable use of parentheses. Defensive use of parentheses, if not taken to excess, also improves code readability. The precedence of operations by the order of the subclauses are defined in the Java Tutorials [[Tutorials 2008]].
The guideline EXP09-J. Do not depend on operator precedence while using expressions containing side-effects advises against depending on parentheses for specifying the evaluation order; however this advice is applicable only to expressions that contain side-effects.
Noncompliant Code Example
The intent of the expression in this noncompliant code example is to add the variable OFFSET
with the result of the bitwise
AND
between x
and MASK
.
public static final int MASK = 1337; public static final int OFFSET = -1337; public static int computeCode(int x) { return x & MASK + OFFSET; }
According to the operator precedence rules, the expression is parsed as the following:
x & (MASK + OFFSET)
This expression gets evaluated as shown below, resulting in the value 0.
x & (1337 - 1337)
Compliant Solution
In this compliant solution, parentheses are used to ensure that the expression evaluates as expected.
public static final int MASK = 1337; public static final int OFFSET = -1337; public static int computeCode(int x) { return (x & MASK) + OFFSET; }
Exceptions
EXP00-EX1: Mathematical expressions that follow algebraic order do not require parentheses. For instance, consider the expression:
x + y * z
By mathematical convention, multiplication is performed before addition. Consequently, parentheses may prove to be redundant in this case.
x + (y * z)
Risk Assessment
Mistakes regarding precedence rules may cause an expression to be evaluated in an unintended way. This can lead to unexpected and abnormal program behavior.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP06-J |
low |
probable |
medium |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Coding Standard as EXP00-C. Use parentheses for precedence of operation.
This rule appears in the C++ Secure Coding Standard as EXP00-CPP. Use parentheses for precedence of operation.
Bibliography
[[Tutorials 2008]] Expressions, Statements, and Blocks, Operators
[[ESA 2005]] Rule 65: Use parentheses to explicitly indicate the order of execution of numerical operators
EXP05-J. Be aware of integer promotions in binary operators 04. Expressions (EXP) EXP07-J. Be aware of the short-circuit behavior of the conditional AND and OR operators