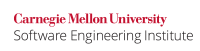
Choosing to implement the Comparable
interface represents a commitment that the implementation of the compareTo()
method adheres to the general contract for that method regarding how the method is to be called. Library classes such as TreeSet
and TreeMap
accept Comparable
objects and use the associated compareTo()
methods to sort the objects. However, a class that implements the compareTo()
method in an unexpected way can cause undesirable results.
The general contract for compareTo()
from Java SE 6 API [API 2006] states that
- The implementor must ensure
sgn(x.compareTo(y)) == -sgn(y.compareTo(x))
for allx
andy
. (This implies thatx.compareTo(y)
must throw an exception ify.compareTo(x)
throws an exception.)- The implementor must also ensure that the relation is transitive:
(x.compareTo(y) > 0 && y.compareTo(z) > 0)
impliesx.compareTo(z) > 0
.- Finally, the implementor must ensure that
x.compareTo(y) == 0
implies thatsgn(x.compareTo(z)) == sgn(y.compareTo(z))
for allz
.- It is strongly recommended, but not strictly required, that
(x.compareTo(y) == 0) == x.equals(y)
. Generally speaking, any class that implements the Comparable interface and violates this condition should clearly indicate this fact. The recommended language is Note: this class has a natural ordering that is inconsistent with equals.In the foregoing description, the notation
sgn(expression)
designates the mathematical signum function, which is defined to return either -1, 0, or 1 depending on whether the value of the expression is negative, zero or positive.
Implementations must never violate any of the first three conditions when implementing the compareTo()
method. Implementations should conform to the fourth condition whenever possible.
Noncompliant Code Example (Rock-Paper-Scissors)
This program implements the classic game of rock-paper-scissors, using the compareTo()
operator to determine the winner of a game.
class GameEntry implements Comparable { public enum Roshambo {ROCK, PAPER, SCISSORS} private Roshambo value; public GameEntry(Roshambo value) { this.value = value; } public int compareTo(Object that) { if (!(that instanceof Roshambo)) { throw new ClassCastException(); } GameEntry t = (GameEntry) that; return (value == t.value) ? 0 : (value == Roshambo.ROCK && t.value == Roshambo.PAPER) ? -1 : (value == Roshambo.PAPER && t.value == Roshambo.SCISSORS) ? -1 : (value == Roshambo.SCISSORS && t.value == Roshambo.ROCK) ? -1 : 1; } }
However, this game violates the required transitivity property because rock beats scissors, scissors beats paper, but rock does not beat paper.
Compliant Solution (Rock-Paper-Scissors)
This compliant solution implements the same game without using the Comparable
interface.
class GameEntry { public enum Roshambo {ROCK, PAPER, SCISSORS} private Roshambo value; public GameEntry(Roshambo value) { this.value = value; } public int beats(Object that) { if (!(that instanceof Roshambo)) { throw new ClassCastException(); } GameEntry t = (GameEntry) that; return (value == t.value) ? 0 : (value == Roshambo.ROCK && t.value == Roshambo.PAPER) ? -1 : (value == Roshambo.PAPER && t.value == Roshambo.SCISSORS) ? -1 : (value == Roshambo.SCISSORS && t.value == Roshambo.ROCK) ? -1 : 1; } }
Risk Assessment
Violating the general contract when implementing the compareTo()
method can result in unexpected results, possibly leading to invalid comparisons and information disclosure.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MET10-J | medium | unlikely | medium | P4 | L3 |
Automated Detection
Automated detections of violations of this rule is infeasible in the general case.
Tool | Version | Checker | Description |
---|---|---|---|
Coverity | 7.5 | FB.RU_INVOKE_RUN | Implemented |
Related Guidelines
CWE-573. Improper following of specification by caller |
Bibliography