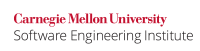
Because an exception is caught by its type, it is better to define exceptions for specific purposes rather than use the general exception types for a variety of different purposes. Throwing the general exception types makes code hard to understand and maintain, and defeats much of the advantage of the Java exception-handling mechanism.
Non-Compliant Code Example
This noncompliant code example attempts to distinguish between different exceptional behavior by looking at the exception's message.
try { doSomething(); } catch(Exception e) { String msg = e.getMessage(); switch (msg) { case "stack underflow": // ... break; case "connection timeout": // ... break; case "security violation": // ... break; default throw e; } }
If doSomething
throws an exception, whose type is a subclass of Exception
, with message "stack underflow" then the appropriate action will be taken in the exception handler. However, any change to the literals involved will break the code. For example, if a maintainer should edit the throw expression to read throw Exception("Stack Underflow");
the exception will be rethrown by the code of the noncompliant code example rather than handled. Also, an exception may be thrown with no message.
Compliant Solution
It is better to user specific existing exception types, or define new, special purpose exception types.
public class StackUnderflowException extends Exception { StackUnderflowException () { super(); } StackUnderflowException (String msg) { super(msg); } } // ... try { doSomething(); } catch(StackUnderflowException sue) { // ... } catch(TimeoutException te) { // ... } catch(SecurityException se) { // ... } catch(Exception e) { // ... throw e; }
Risk Assessment
Exceptions are used to handle exceptional conditions. If an exception is not caught, the program will be terminated. An exception that is incorrectly caught, or caught at the wrong level of recovery will likely cause incorrect behavior.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ERR54-J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
Automated detection is not feasible.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Other Languages
This guideline appears in the C++ Secure Coding Standard as ERR08-CPP. Prefer special-purpose types for exceptions.
Bibliography
[[JLS 2011]] Chapter 11. Exceptions