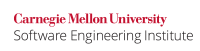
Objects of a class can be ordered relative to one another. One way to do this is for the class to implement the Comparable
interface. Library classes such as TreeSet
and TreeMap
accept Comparable
objects and use their compareTo()
methods to sort them. However, a class that implements the compareTo()
method in an unexpected way can cause undesirable results.
The general usage contract for compareTo()
from Java SE 6 API [[API 06]] states that:
The implementor must ensure
sgn(x.compareTo(y)) == -sgn(y.compareTo(x))
for all x and y. (This implies thatx.compareTo(y)
must throw an exception iffy.compareTo(x)
throws an exception.)The implementor must also ensure that the relation is transitive:
(x.compareTo(y) >0 && y.compareTo(z)>0)
impliesx.compareTo(z)>0
.Finally, the implementor must ensure that
x.compareTo(y) ==0
implies thatsgn(x.compareTo(z)) == sgn(y.compareTo(z))
, for allz
.It is strongly recommended, but not strictly required that
(x.compareTo(y) ==0) == (x.equals(y) )
. Generally speaking, any class that implements the Comparable interface and violates this condition should clearly indicate this fact. The recommended language is "Note: this class has a natural ordering that is inconsistent with equals."In the foregoing description, the notation
sgn(expression)
designates the mathematical signum function, which is defined to return either -1, 0, or 1 depending on whether the value of the expression is negative, zero or positive.
Do not violate any of the first three conditions when implementing the compareTo()
method. Implementing the fourth condition is strongly recommended but is not necessary.
Noncompliant Code Example
This noncompliant code example violates the third condition (transitivity) in the contract. This requirement states that the objects that compareTo()
considers equal (returns 0) must be ordered the same with respect to other objects. For example, a card is to be compared against any other card to check whether both belong to the same suit or have the same rank. If neither of these conditions is true, compareTo()
is expected to order the cards based on rank alone. This might arise in a game like Uno or Crazy Eights, where you can only place a card on the pile that shares a suit or rank with the top card on the pile.
public final class Card implements Comparable { private String suit; private int rank; public Card(String s, int r) { if (s == null) throw new NullPointerException(); suit = s; rank = r; } public boolean equals(Object o) { if (o instanceof Card) { Card c = (Card)o; return suit.equals(c.suit) || (rank == c.rank); // bad } return false; } // This method violates its contract public int compareTo(Object o) { if (o instanceof Card) { Card c = (Card)o; if( suit.equals(c.suit) ) return 0; if( (c.rank >= rank + Integer.MIN_VALUE) && (c.rank <= rank + Integer.MAX_VALUE) ) // check for integer underflow/overflow return c.rank - rank; // order based on rank } throw new ClassCastException(); } public static void main(String[] args) { Card a = new Card("Clubs", 2); Card b = new Card("Clubs", 10); Card c = new Card("Hearts", 7); System.out.println(a.compareTo(b)); // returns 0 System.out.println(a.compareTo(c)); // returns a negative number System.out.println(b.compareTo(c)); // returns a positive number } }
Here, the comparison between (a,c)
yields that c
is larger. However, the comparison (b,c)
yields b
as larger. This means b
must be larger than a
. However, comparing (a,b)
results in the value 0
(same).
Compliant Solution
This compliant solution ensures that the compareTo()
contract is satisfied, and the corresponding equals()
method is consistent with compareTo()
.
public final class Card implements Comparable{ private String suit; private int rank; public Card(String s, int r) { if (s == null) throw new NullPointerException(); suit = s; rank = r; } public boolean equals(Object o) { if (o instanceof Card) { Card c=(Card)o; return suit.equals(c.suit) && (rank == c.rank); // good } return false; } // this method fulfills its contract public int compareTo(Object o) { if (o instanceof Card) { Card c=(Card)o; if( suit.equals(c.suit) && (c.rank >= rank + Integer.MIN_VALUE) && (c.rank <= rank + Integer.MAX_VALUE) ) return c.rank - rank; return suit.compareTo(c.suit); } throw new ClassCastException(); } public static void main(String[] args) { Card a = new Card("Clubs", 2); Card b = new Card("Clubs", 10); Card c = new Card("Hearts", 7); System.out.println(a.compareTo(b)); // returns 0 System.out.println(a.compareTo(c)); // returns a negative number System.out.println(b.compareTo(c)); // returns a negative number } }
As required by the ordering, c
is larger than both a
and b
and the comparison (a,b)
produces an equal result. This maintains the compareTo
contract.
Risk Assessment
Violating the general contract when implementing the compareTo()
method can lead to unexpected results, possibly leading to invalid comparisons and information disclosure.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET34- J |
medium |
unlikely |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as ARR40-CPP. Use a Valid Ordering Rule.
References
[[API 06]] method compareTo()
[[JLS 05]]
MET33-J. Do not subject overloaded methods to polymorphic invocations 10. Methods (MET) MET35-J. Ensure that the clone method calls super.clone