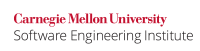
Files on multiuser systems are generally owned by a particular user. The owner of the file can determine which other users on the system should be allowed to access the contents of these files.
These file systems generally use a privileges and permissions model to protect file access. When a file is created, the access permissions of the file immediately dictate who may access or operate on the file. If a program creates a file with insufficiently restrictive access permissions, an attacker may read or modify the file before the program can modify the permissions. Consequently, files must be created with access permissions that prevent unauthorized file access.
Noncompliant Code Example
The constructors for FileOutputStream
and FileWriter
do not allow the programmer to explicitly specify file access permissions.
In this noncompliant code example, the access permissions of any file created is implementation-defined and may fail to prevent unauthorized access.
Writer out = new FileWriter("file");
Compliant Solution
Java 1.6 and earlier provide no mechanism for specifying default permissions upon file creation. Consequently, the problem must be avoided or solved using some mechanism external to Java, such as by using native code and JNI.
Compliant Solution (Java 1.7, POSIX)
The Java 1.7 new I/O facility (java.nio
) provides classes for managing file access permissions. Additionally, many of the methods and constructors that create files accept an argument allowing the program to specify the initial file permissions.
The Files.newByteChannel()
method allows a file to be created with specific permissions. This method is platform-independent, but the actual permissions are platform-specific. The following compliant solution shows sufficiently restrictive permissions for POSIX platforms.
Path file = new File("file").toPath(); // Throw exception rather than overwrite existing file Set<OpenOption> options = new HashSet<OpenOption>(); options.add(StandardOpenOption.CREATE_NEW); options.add(StandardOpenOption.APPEND); // File permissions should be such that only user may read/write file Set<PosixFilePermission> perms = PosixFilePermissions.fromString("rw-------"); FileAttribute<Set<PosixFilePermission>> attr = PosixFilePermissions.asFileAttribute(perms); try (SeekableByteChannel sbc = Files.newByteChannel(file, options, attr)) { // write data };
Exceptions
FIO03-EX0: If a file is created inside a directory that is both secure and unreadable by untrusted users, then that file may be created with the default access permissions. See FIO03-J. Remove temporary files before termination for the definition of a secure directory. This could be the case if, for example, the entire file system is trusted, or is accessible only to trusted users.
FIO03-EX1: Files that do not contain sensitive information need not be created with specific access permissions.
Risk Assessment
The ability to determine if an existing file has been opened or a new file has been created provides greater assurance that a file other than the intended file is not acted upon.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO03-J |
medium |
probable |
high |
P4 |
L3 |
Automated Detection
TODO
Related Guidelines
CWE-279, "Incorrect Execution-Assigned Permissions" |
|
|
CWE-276, "Incorrect Default Permissions" |
|
CWE-732, "Incorrect Permission Assignment for Critical Resource" |
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="e96674eb-e815-4b8f-ac4d-b193b7f4037f"><ac:plain-text-body><![CDATA[ |
[[API 2006 |
AA. Bibliography#API 06]] |
|
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="39e7637d-c2ae-4062-80bc-61ad3c3e173d"><ac:plain-text-body><![CDATA[ |
[[CVE |
AA. Bibliography#CVE]] |
|
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="ca822234-8497-4cbf-82e0-a1079b9b6a29"><ac:plain-text-body><![CDATA[ |
[[Dowd 2006 |
AA. Bibliography#Dowd 06]] |
Chapter 9, "UNIX 1: Privileges and Files" |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="f6e75894-fbf9-4c64-a032-b43eb25dfe5c"><ac:plain-text-body><![CDATA[ |
[[J2SE 2011 |
AA. Bibliography#J2SE 11]] |
|
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="a7db6d7c-0b3b-49f5-90dd-1b3e5caf66b1"><ac:plain-text-body><![CDATA[ |
[[OpenBSD |
AA. Bibliography#OpenBSD]] |
|
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="5e7eb0ce-c901-4ddf-b475-dcc8b2c0b141"><ac:plain-text-body><![CDATA[ |
[[Open Group 2004 |
AA. Bibliography#Open Group 04]] |
"The |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="444a8739-1a00-4044-8b22-bf3eddfce8d1"><ac:plain-text-body><![CDATA[ |
[[Viega 2003 |
AA. Bibliography#Viega 03]] |
Section 2.7, "Restricting Access Permissions for New Files on UNIX" |
]]></ac:plain-text-body></ac:structured-macro> |