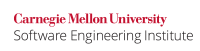
According to the Java API [[API 2006]], class java.io.File
A path name, whether abstract or in string form, may be either absolute or relative. An absolute path name is complete in that no other information is required to locate the file that it denotes. A relative path name, in contrast, must be interpreted in terms of information taken from some other path name.
An absolute path may contain aliases, shadows, symbolic links and shortcuts (aliases, hereafter) rather than canonical paths, which refer to the actual files or directories that these point to. These aliases must be fully resolved before any file validation operations are performed. For instance, resolving a symbolic link called trace
may yield its actual path on the file system, such as, /home/system/trace
.
The process of canonicalizing file names makes it easier to verify an alias. More than one alias can refer to a single directory or file. Further, the textual representation of an alias may yield little or no information regarding the directory or file to which it refers. Consequently, all aliases must be fully resolved or canonicalized before validation. This is necessary because untrusted user input may allow an I/O operation to escape the specified operating directory. Violation of this rule can result in information disclosure and malicious modification of files existing in directories other than the specified one.
This rule is an instance of IDS02-J. Normalize strings before validating them.
Noncompliant Code Example
This noncompliant code example accepts a file path as a command line argument and uses the File.getAbsolutePath()
method to obtain the absolute file path. This method does not automatically resolve symbolic links.
public static void main(String[] args) { File f = new File("/tmp/" + args[0]); String absPath = f.getAbsolutePath(); if (!validate(absPath)) { // Validation throw new IllegalArgumentException(); } }
The application intends to restrict the user from operating on files outside the /tmp
directory and uses a validate()
method to enforce this condition. An attacker who can create symbolic links in /tmp
can cause the program to pass validation checks by supplying the unresolved path. All file operations performed are reflected in the file pointed to by the symbolic link.
Let argv[0]
be the string filename
, where /tmp/filename
is a symbolic link that points to the file /dirname/filename
present on the local file system. The validation passes because the root directory of the compiled path name is still /tmp
, but the operations are carried out on the file /dirname/filename
.
Note that File.getAbsolutePath()
actually does resolve all symbolic links, aliases and short cuts on Windows and Macintosh platforms. Nevertheless, the JLS lacks any guarantee that this behavior is present on all platforms or that it will continue in future implementations.
Compliant Solution (getCanonicalPath()
)
This compliant solution uses the getCanonicalPath()
method, introduced in Java 2, because it resolves all aliases, shortcuts or symbolic links consistently, across all platforms. The value of the alias (if any) is not included in the returned value. Moreover, relative references like the double period (..
) are also removed so that the input is reduced to a canonicalized form before validation is carried out. An attacker cannot use ../
sequences to break out of the specified directory when the validate()
method is present.
public static void main(String[] args) throws IOException { File f = new File("/tmp/" + args[0]); String canonicalPath = f.getCanonicalPath(); if (!validate(canonicalPath)) { // Validation throw new IllegalArgumentException(); } }
The getCanonicalPath()
method throws a security exception when used within applets as it reveals too much information about the host machine. The getCanonicalFile()
method behaves like getCanonicalPath()
but returns a new File
object instead of a String
.
Compliant Solution (Security Manager)
A comprehensive way of handling this issue is to grant the application the permissions to operate only on files present within the intended directory — /tmp
in this example. This compliant solution specifies the absolute path of the program in its security policy file, and grants java.io.FilePermission
with target /tmp
and actions read
and write
.
grant codeBase "file:/home/programpath/" { permission java.io.FilePermission "/tmp", "read, write"; };
See rule ENV02-J. Create a secure sandbox using a Security Manager for additional information on using security managers.
Noncompliant Code Example
This noncompliant code example allows the user to specify the absolute path of a file name on which to operate. The user can specify files outside the intended directory (/img
in this example) by entering an argument that contains ../
sequences, and consequently violate the intended security policies of the program.
FileOutputStream fis = new FileOutputStream(new File("/img/" + args[0])); // ...
Noncompliant Code Example
This noncompliant code example attempts to mitigate the issue by using the File.getCanonicalPath()
method, which fully resolves the argument and constructs a canonicalized path. For example, the path /img/../etc/passwd
resolves to /etc/passwd
. Validation without canonicalization remains insecure because the user can specify files outside the intended directory.
File f = new File("/img/" + args[0]); String canonicalPath = f.getCanonicalPath(); FileOutputStream fis = new FileOutputStream(f); // ...
Compliant Solution
This compliant solution obtains the file name from the untrusted user input, canonicalizes it and then validates it against the intended file name. It operates on the specified file only when validation succeeds.
File f = new File("/img/" + args[0]); String canonicalPath = f.getCanonicalPath(); if (canonicalPath.equals("/img/java/file1.txt")) { // Validation // Do something } if (!canonicalPath.equals("/img/java/file2.txt")) { // Validation // Do something } FileOutputStream fis = new FileOutputStream(f);
Compliant solution (Security Manager)
A comprehensive solution is to grant the application the permissions to read only the specifically intended files or directories. Grant these permissions by to specifying the absolute path of the program in the security policy file and granting java.io.FilePermission
with the canonicalized absolute path of the file or directory as the target name and with the action set to read
.
// All files in /img/java can be read grant codeBase "file:/home/programpath/" { permission java.io.FilePermission "/img/java", "read"; };
See rule ENV02-J. Create a secure sandbox using a Security Manager for additional information on using security managers.
Risk Assessment
Using path names from untrusted sources without first canonicalizing them and then validating them can result in directory traversal attacks.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
IDS21-J |
medium |
unlikely |
medium |
P4 |
L3 |
Other Languages
This rule appears in the C Secure Coding Standard as FIO02-C. Canonicalize path names originating from untrusted sources.
This rule appears in the C++ Secure Coding Standard as FIO02-CPP. Canonicalize path names originating from untrusted sources.
Related Vulnerabilities
|
||||
|
||||
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="41727566-164b-49b4-a1f3-a53b9875184e"><ac:plain-text-body><![CDATA[ |
[[MITRE 2009 |
AA. Bibliography#MITRE 09]] |
[CWE ID 171 |
http://cwe.mitre.org/data/definitions/171.html] "Cleansing, Canonicalization, and Comparison Errors"]]></ac:plain-text-body></ac:structured-macro> |
|
CWE ID 647 "Use of Non-Canonical URL Paths for Authorization Decisions" |
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="bd6ba6e0-1548-4bcc-a9ad-c2fdff646163"><ac:plain-text-body><![CDATA[ |
[[API 2006 |
AA. Bibliography#API 06]] |
[method getCanonicalPath() |
http://java.sun.com/javase/6/docs/api/java/io/File.html#getCanonicalPath()] |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="0fc24b6e-da54-4c84-a314-32486aff0a75"><ac:plain-text-body><![CDATA[ |
[[API 2006 |
AA. Bibliography#API 06]] |
[method getCanonicalFile() |
http://java.sun.com/javase/6/docs/api/java/io/File.html#getCanonicalFile()] |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="e60cab26-75ea-4108-b1c1-8dd6cb3a8e67"><ac:plain-text-body><![CDATA[ |
[[Harold 1999 |
AA. Bibliography#Harold 99]] |
|
]]></ac:plain-text-body></ac:structured-macro> |
IDS10-J. Exclude user input from format strings IDS06-J. Limit the size of files passed to ZipInputStream